Whether you’re browsing the web, sending a message, or playing an online game, sockets are the underlying technology enabling devices to communicate over a network.
Table of Contents
What is a Socket?
A socket is an endpoint for sending or receiving data across a computer network. Think of it as a two-way communication pipe connecting two programs—one running on your machine, and one on another (or the same) machine.
Each socket is bound to an IP address and a port number:
- IP Address = The unique address of a device on a network.
- Port Number = A specific channel on that device used for communication.
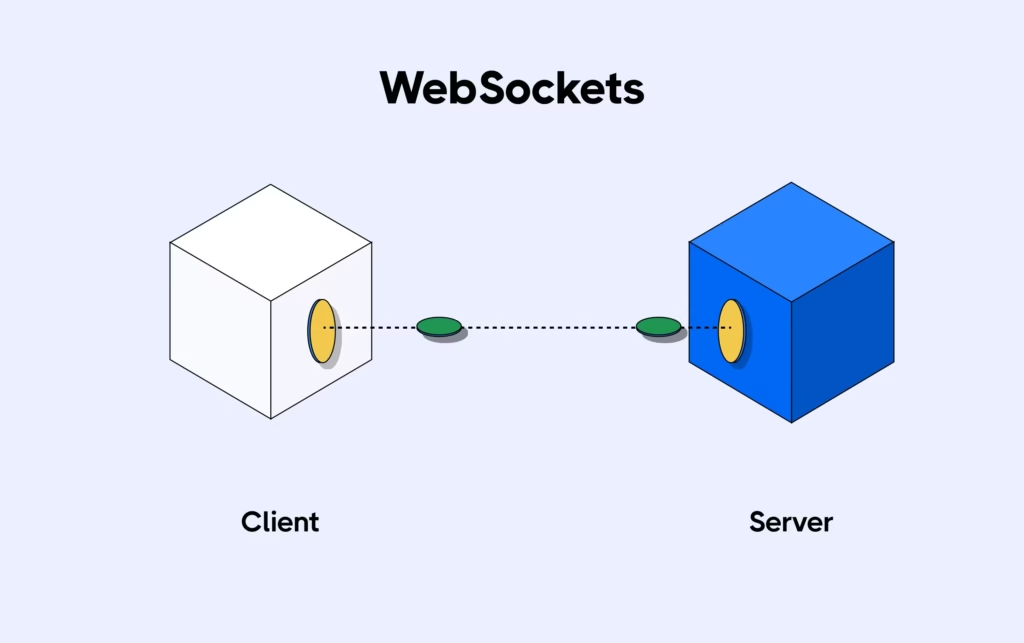
How Do Sockets Work?
Here’s a simple flow of a typical socket communication:
Server side:
- Create a socket.
- Bind it to an IP address and port.
- Listen for incoming connections.
- Accept connections and communicate.
Client side:
- Create a socket.
- Connect to the server’s IP and port.
- Send/receive data.
The communication can use:
- TCP (Transmission Control Protocol) – reliable, connection-oriented.
- UDP (User Datagram Protocol) – faster, connectionless.
Real-World Analogy
Imagine a phone call:
- You dial someone’s number (connect to IP and port).
- Once connected, both can speak and listen (send/receive data).
- When the call ends, the line is closed (socket is closed).
That’s essentially how socket communication happens!
Types of Sockets
Type | Description |
---|---|
Stream Socket (TCP) | Provides reliable, ordered, and error-checked delivery of data. |
Datagram Socket (UDP) | Sends packets without a connection, faster but less reliable. |
Raw Socket | Used for low-level access to the network, often by system utilities or network tools. |
Python Socket Programming: A Simple Example
Let’s build a basic TCP chat between a server and a client.
Server Code
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(1)
print("Server is waiting for connection...")
conn, addr = server_socket.accept()
print(f"Connected by {addr}")
while True:
data = conn.recv(1024).decode()
if not data or data.lower() == 'bye':
break
print("Client:", data)
reply = input("You: ")
conn.send(reply.encode())
conn.close()
PythonClient Code
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 12345))
while True:
message = input("You: ")
client_socket.send(message.encode())
if message.lower() == 'bye':
break
reply = client_socket.recv(1024).decode()
print("Server:", reply)
client_socket.close()
PythonThings to Keep in Mind
- Always close sockets after use (socket.close()).
- Use try-except blocks to handle connection errors.
- Don’t hard-code IPs and ports in production—use environment configs.
Real-Life Applications of Sockets
- Web servers (e.g., Apache, Nginx)
- Chat apps (WhatsApp, Messenger)
- Online games (Multiplayer communication)
- IoT devices (Remote monitoring and control)
- File transfer apps (FTP, SCP)
Persistent Socket Connection
A persistent connection (also known as a keep-alive connection) keeps the communication channel open after the initial data transfer, allowing further communication without the overhead of establishing new connections every time.
Why Use Sockets for Persistent Connections?
Using sockets for persistent connections is beneficial for:
Real-time Communication
- Messaging apps (e.g., WhatsApp, Slack)
- Live chats or customer support systems
Streaming Services
- Video/audio streaming
- Real-time data feeds (e.g., stock prices, sports scores)
Online Gaming
- Multiplayer games use persistent socket connections to sync player actions in real-time.
IoT Devices
- Continuous communication between devices and servers.
Remote Procedure Calls (RPC)
- Systems that invoke procedures on remote systems often use persistent socket connections.
Advantages
- Reduces latency by avoiding repeated handshakes.
- Lowers the overhead on both client and server.
- Enables real-time bidirectional data flow.
- Improves efficiency in applications requiring frequent communication.
Considerations
- Needs proper timeout handling and error detection.
- Must manage connection drops and retries.
- Servers need to handle concurrent connections efficiently (e.g., using threading, asyncio, or event-driven frameworks).
How It Works (TCP Example)
A typical TCP socket connection lifecycle with persistence:
Connection Establishment
- socket.connect() on the client
- socket.accept() on the server
Data Transfer
- Both client and server use send() and recv() to exchange data.
Persistent Communication
- Instead of closing the connection, both ends keep it open and continue reading/writing data.
Connection Termination
- Only after all necessary data is exchanged, the connection is closed using socket.close().
Conclusion
Sockets may seem low-level, but they are the glue holding together most of the internet. Mastering socket programming can give you a powerful edge when building anything from backend services to real-time applications.
Whether you’re debugging, building apps, or exploring networking, understanding sockets is a must for any serious developer.