APIs (Application Programming Interfaces) play a central role in enabling communication between software systems. From retrieving weather data to enabling online payments, APIs act as bridges between different applications. Whether you’re building a mobile app, web service, or enterprise solution, understanding APIs is crucial.
Table of Contents
What is an API?
An API is a set of rules and protocols that allows one piece of software or program to interact with another.
Think of it as a contract between two software components — the API defines how requests and responses should be structured, without exposing the internal workings of a system.
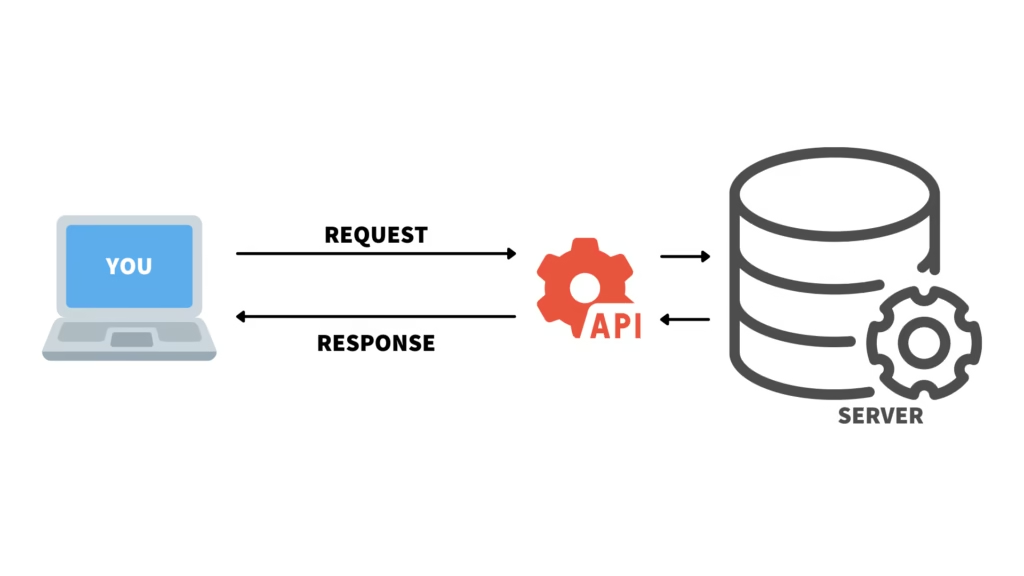
Real-world Analogy:
Imagine a restaurant:
- You are the client.
- The kitchen is the server.
- The waiter is the API — taking your request (order), delivering it to the kitchen, and bringing the response (your food).
Components of an API
Endpoint
- The URL path where the API can be accessed (e.g., https://api.example.com/users).
Request
- Method (GET, POST, PUT, DELETE)
- Headers (Authorization, Content-Type)
- Body (optional data for POST/PUT)
Response
- Status code (e.g., 200 OK, 404 Not Found)
- Headers (metadata about the response)
- Body (usually in JSON, XML)
Resources
- The objects (like users, orders, products) the API interacts with.
Types of APIs
API Type | Description | Examples |
---|---|---|
Open/Public | Available for use by developers and users with minimal restrictions | GitHub API, Twitter API |
Partner | Shared with specific partners under controlled access | Shopify Partner API |
Private | Used internally within an organization | Internal microservices API |
Composite | Combines multiple services into a single call | Aggregation APIs in microservices |
API Protocols and Architectures
REST (Representational State Transfer)
Most widely used; stateless; uses HTTP.
- Methods: GET, POST, PUT, DELETE
- Data Format: JSON or XML
Example:
GET https://api.example.com/users/42
PythonSOAP (Simple Object Access Protocol)
- XML-based protocol
- Heavier and more secure
- Ideal for enterprise-grade apps
- Example: WSDL-based services
GraphQL
- Developed by Facebook
- Clients can specify exactly what data they need
- Single endpoint
Example Query:
{
user(id: "42") {
name
email
}
}
PythongRPC (Google Remote Procedure Call)
- Uses Protocol Buffers (protobuf)
- High performance, ideal for microservices
- Strongly typed
API Authentication & Authorization
Security is critical for APIs. Common authentication methods include:
Method | Description |
---|---|
API Key | Simple token passed in headers or URL |
OAuth 2.0 | Authorization framework, often used with 3rd parties |
JWT (JSON Web Token) | Secure tokens that carry authentication info |
Basic Auth | Username/password encoded in base64 |
HTTP Methods in RESTful APIs
Method | Description | Example Use Case |
---|---|---|
GET | Retrieve data | GET /products |
POST | Create new resource | POST /orders |
PUT | Update existing resource (full) | PUT /users/123 |
PATCH | Partial update | PATCH /users/123 |
DELETE | Remove a resource | DELETE /posts/50 |
Status Codes (HTTP Response)
Code | Meaning | Description |
---|---|---|
200 | OK | Successful request |
201 | Created | Resource successfully created |
400 | Bad Request | Client-side input error |
401 | Unauthorized | Authentication required |
403 | Forbidden | Not allowed |
404 | Not Found | Resource doesn’t exist |
500 | Internal Server Error | Something went wrong on server |
Best Practices for API Design
- Use RESTful conventions
- Version your APIs (e.g., /api/v1/users)
- Use meaningful resource names (/users, not /getUsers)
- Paginate large results (e.g., ?page=2&limit=20)
- Rate limiting and throttling to prevent abuse
- Clear error messages with details and codes
- Secure sensitive data using HTTPS and OAuth/JWT
- Provide good documentation (Swagger, Postman, etc.)
API Testing Tools
Tool | Description |
---|---|
Postman | GUI for sending API requests |
Insomnia | Similar to Postman, lightweight |
cURL | Command-line tool |
Swagger | API documentation and interactive docs |
JMeter | Load testing and performance testing |
API Documentation and Specification Tools
- Swagger / OpenAPI – Design and document REST APIs.
- RAML – RESTful API Modeling Language.
- API Blueprint – Markdown-based documentation.
Conclusion
APIs are the building blocks of modern software. Whether you’re consuming third-party services or exposing your own, understanding APIs enables scalability, integration, and automation. Mastering them opens doors to endless possibilities — from building microservices to integrating machine learning services.
2 thoughts on “Understanding APIs: A Comprehensive Guide”