As software development increasingly embraces multi-threading for improved performance, ensuring thread safety becomes essential. Thread safety guarantees that multiple threads can safely access shared resources without causing data corruption or unpredictable behaviour. In this article, we’ll explore the importance of thread safety and practical strategies for implementing it in your applications.
Table of Contents
What is Thread Safety?
Thread safety is a property of a program or a data structure that guarantees safe execution by multiple threads at the same time without unintended interactions. When a piece of code is thread safe, it ensures that the results of its execution are the same, regardless of how many threads are executing the code concurrently.
Example: Consider a scenario where multiple threads attempt to increment a shared counter. If one thread reads the counter’s value while another thread updates it, inconsistencies can arise, leading to incorrect results. A thread-safe counter would manage this situation, ensuring that all updates are done without interference.
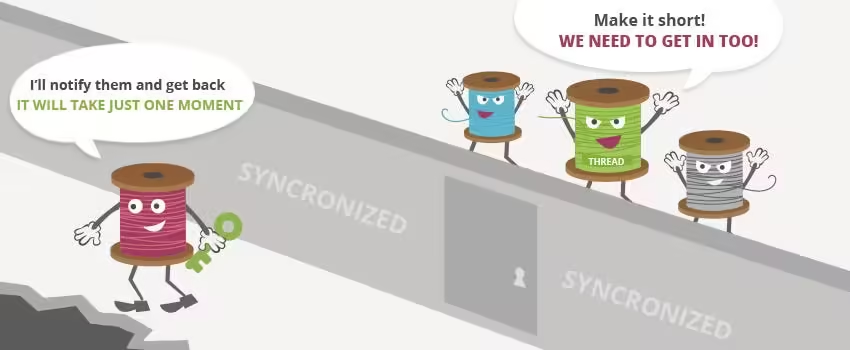
Why is Thread Safety Important?
- Data Integrity: In a multi-threaded environment, shared data can be modified by multiple threads simultaneously. Thread safety prevents data corruption and maintains data integrity.
- Predictable Behavior: Ensuring that code is thread-safe helps avoid unpredictable behaviour caused by race conditions, deadlocks, or livelocks, which can be challenging to debug.
- Scalability: Thread-safe code can be scaled to leverage multi-core processors, improving the performance and responsiveness of applications without compromising reliability.
Achieving Thread-Safety
There are several techniques that developers can employ to achieve thread safety:
- Mutexes and Locks: Mutex (Mutual Exclusion): A mutex is a synchronization primitive that prevents multiple threads from accessing a resource simultaneously. By locking a mutex before accessing shared data, developers can ensure that only one thread can modify the data at a time.
Python Example
import threading
counter = 0
lock = threading.Lock()
def increment():
global counter
with lock:
counter += 1
threads = [threading.Thread(target=increment) for _ in range(100)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
print(counter) # Output will reliably be 100
Python- Atomic Operations: Some programming languages provide atomic operations that guarantee operations on variables are completed in a single step without interruption. These are often faster than using locks.
- Example: In C++,
std::atomic
provides atomic operations for built-in types.
- Example: In C++,
- Read-Write Locks: When a resource is read frequently but updated infrequently, read-write locks allow multiple threads to read simultaneously while providing exclusive access for writing.
- Thread-Local Storage: This technique allows each thread to have its instance of a variable, eliminating the need for synchronization since no two threads can access the same instance.
- Immutability: Designing data structures to be immutable can also enhance thread safety. Once created, immutable objects cannot be altered, which means they can be shared between threads without risk of modification.
Challenges of Thread-Safety
While implementing thread-safety is essential, it comes with its challenges:
- Performance Overhead: Locking mechanisms can introduce latency and reduce performance, particularly if they lead to contention among threads.
- Complexity: Writing thread-safe code can increase the complexity of the codebase, making it harder to read and maintain.
- Deadlocks: Improper use of locks can lead to deadlocks, where two or more threads wait indefinitely for resources held by each other.
Conclusion
Thread safety is a vital aspect of software development in a multi-threaded environment. By understanding its importance and employing techniques such as locks, atomic operations, and immutability, developers can ensure that their applications run reliably and efficiently. As multi-core processors become increasingly common, mastering thread safety will be essential for building robust, high-performance software.
1 thought on “Thread Safety: Safeguarding Your Code in a Multi-Threaded World”