Gunicorn, short for “Green Unicorn,” is a Python WSGI (Web Server Gateway Interface) HTTP server for UNIX. It is a widely used and well-regarded production server that allows developers to run Python web applications. Its lightweight nature, ease of configuration, and performance make it the go-to server for many Python web applications, especially those built on popular frameworks like Flask and Django. In this article, we’ll explore what Gunicorn is, how it works, and why it is widely adopted.
Table of Contents
What is Gunicorn?
Gunicorn is a Python HTTP server for running web applications using the WSGI standard. It acts as a bridge between web clients (such as browsers) and Python web applications.
By handling HTTP requests and passing them to Python applications, it allows developers to focus on building features without worrying about the complexities of serving HTTP requests directly.
Gunicorn is designed to be simple, fast, and lightweight, and it is compatible with various web frameworks that adhere to the WSGI specification.
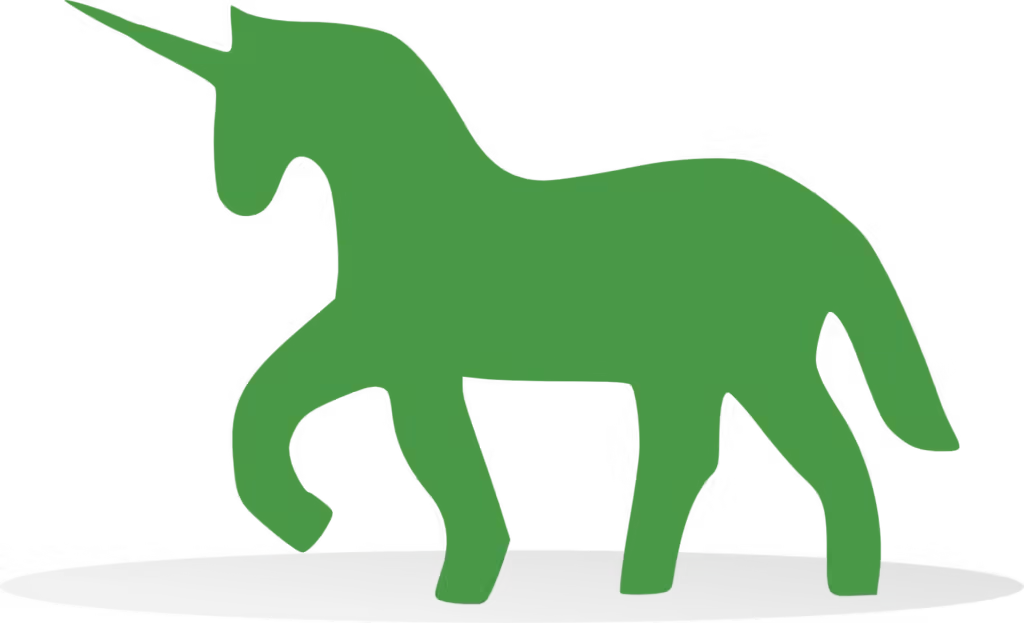
The Role of WSGI in Python Web Development
To understand Gunicorn fully, it’s essential to grasp the role of WSGI. WSGI, or Web Server Gateway Interface, is a specification that defines a standard interface between web servers and Web applications or frameworks. WSGI was created to standardize how web applications interact with servers, enabling interoperability and flexibility.
Without WSGI, each framework or application would have to handle low-level details of HTTP communication differently. Gunicorn and other WSGI-compliant servers abstract this complexity, letting developers use a single, well-defined interface to serve their web applications.
How Gunicorn Works
Gunicorn is based on a pre-fork worker model, inspired by the traditional UNIX philosophy of spawning child processes to handle work. Here’s a simplified process of how Gunicorn operates:
- Master Process: When Gunicorn starts, it spawns a master process that listens for incoming HTTP requests.
- Worker Processes: The master process forks off worker processes, each of which handles incoming requests. Workers execute the Python web application code (via the WSGI interface) and return the response to the client.
- Concurrency Handling: Gunicorn supports both synchronous and asynchronous workers, allowing developers to scale their applications effectively based on workload demands.
The default worker type is synchronous, but Gunicorn also supports asynchronous workers using libraries like gevent or eventlet.
Key Features of Gunicorn
- Pre-fork Worker Model: This allows for parallel request handling, making Gunicorn capable of scaling efficiently under heavy loads.
- Multiple Worker Types: Gunicorn supports both synchronous and asynchronous workers, offering flexibility depending on the use case.
- Framework Agnostic: Gunicorn can serve any Python web framework that adheres to the WSGI standard, including Flask, Django, Pyramid, and others.
- Simple to Use: Gunicorn can be started with minimal configuration and offers a straightforward command-line interface for common use cases.
- Graceful Worker Restart: Workers can be restarted gracefully without interrupting ongoing requests, ensuring zero downtime during deployments or server changes.
- Supports SSL/TLS: Gunicorn can be configured to support HTTPS connections, ensuring secure communication.
- Hooks and Signals: Gunicorn provides a set of customizable hooks and signal handlers for more granular control over the server lifecycle.
Gunicorn Architecture
Gunicorn follows a simple yet powerful architecture:
- Master Process: The master process is responsible for managing worker processes. It listens for incoming HTTP connections and routes them to workers. If a worker crashes or becomes unresponsive, the master process spawns a new one.
- Worker Processes: Workers are the actual engines that handle requests. Each worker processes one or more requests depending on its concurrency model. Gunicorn supports:
- Sync workers: Suitable for handling typical web workloads with one request per worker.
- Async workers: Designed for handling I/O-bound applications with many simultaneous connections.
- Communication: The master process forwards incoming HTTP requests to idle workers. Workers execute the application code and return responses. This process is efficient and ensures that your application can handle multiple requests concurrently.
Understanding Workers and Threads
Gunicorn’s performance and scalability can be fine-tuned by configuring the number of workers and threads. These settings determine how your application handles multiple requests and can significantly impact its ability to serve traffic efficiently.
- Workers: A worker in Gunicorn is a separate process that handles incoming requests. Each worker processes one or more requests at a time, depending on its concurrency model. By default, Gunicorn spawns synchronous workers, meaning each worker can handle only one request at a time. Increasing the number of workers allows the server to handle more requests in parallel.
- Threads: Threads allow for concurrency within a single worker process. A worker can be configured to handle multiple threads, each capable of managing a separate request. This is particularly useful for applications that perform I/O-bound operations like database queries or HTTP requests.
In a typical scenario, increasing the number of workers ensures that Gunicorn can handle more requests by distributing them across multiple CPU cores. Adding threads helps with managing workloads that involve waiting for I/O operations, allowing one worker to handle multiple connections at once without blocking.
How Many Workers and Threads Should You Use?
The ideal configuration depends on your application’s specific workload and the available server resources. Here are some guidelines for determining the appropriate number of workers and threads:
Choosing the Right Number of Workers
- CPU-Bound Applications: If your application is CPU-intensive (e.g., machine learning inference, complex computations), you should set the number of workers equal to or slightly higher than the number of CPU cores on your server. This ensures that each CPU core is handling a worker, maximizing resource usage.
- I/O-Bound Applications: For applications that spend a lot of time waiting on external resources (e.g., databases, APIs), you may want more workers. Increasing the worker count allows Gunicorn to manage more simultaneous connections, reducing response times during high traffic.
Choosing the Right Number of Threads
- Threading for I/O-Bound Workloads: Applications that are I/O-bound, such as those making database queries or network calls, can benefit from multiple threads per worker. This allows one worker process to handle multiple requests concurrently, even while waiting on I/O operations. A good starting point is to use 2 to 4 threads per worker.
- Avoid Overthreading: While threads can improve concurrency, having too many threads can lead to context-switching overhead and degrade performance. If your application is CPU-bound, it’s often better to increase the number of workers rather than threads.
Configuring workers and threads in Gunicorn is crucial to optimizing your application’s performance. The right balance will depend on the workload characteristics of your application and the resources available on your server. By understanding how workers and threads impact concurrency and resource usage, you can ensure that your Python web application is able to handle high traffic efficiently.
Start with basic settings, monitor your application in production, and make adjustments as needed to achieve optimal performance. With the flexibility of Gunicorn, you can scale your web applications smoothly, ensuring reliability and responsiveness for your users.
Calculating Total Requests
The total number of concurrent requests Gunicorn can handle is approximately:
Total Requests = Number of Workers * Number of Threads per Worker
Example: We have 4 CPU cores with 9 workers and 4 threads per worker
Total Requests = 9 workers * 4 threads per worker
= 36 concurrent requests
Calculating the number of requests per second. Assuming the average request duration is 200ms
Requests per Thread per Second = 1 / Request Duration
= 1 / 0.2
= 5 requests per second
Now, to calculate the total requests per second Gunicorn can handle:
Total Requests per Second = Total Threads * Requests per Thread per Second
= (9 workers * 4 threads per worker) * 5 requests per second
= 36 * 5
= 180 requests per second
Thus, with 9 workers, 4 threads per worker, and an average request duration of 200ms, the server can handle 180 requests per second.
Gunicorn vs. Other Python Web Servers
Gunicorn is often compared to other Python WSGI servers such as uWSGI and Daphne. Here’s a brief comparison:
- Gunicorn vs uWSGI: Gunicorn is known for its simplicity, while uWSGI is more feature-rich but complex. uWSGI has more options for deployment configurations but can be overkill for many applications. Gunicorn’s simpler configuration is preferable for developers who need a fast and stable production server without unnecessary complexity.
- Gunicorn vs Daphne: Daphne is an ASGI server primarily used for asynchronous frameworks like Django Channels. If your application requires asynchronous functionality (WebSockets, long-lived connections), Daphne may be a better choice, though Gunicorn can handle similar use cases when configured with async workers.
Common Use Cases and Best Practices
- Scaling Applications: Gunicorn’s multi-worker architecture helps scale applications across multiple cores on a server.
- Asynchronous Workloads: By configuring async workers with gevent or eventlet, Gunicorn can handle thousands of concurrent connections efficiently.
- Deploying Microservices: Gunicorn is lightweight and performs well in microservices architectures where simple and effective HTTP handling is required.
- Secure Applications: Use Gunicorn with SSL/TLS to secure communication, especially when deploying sensitive web applications.
Conclusion
Gunicorn is a powerful and flexible WSGI server that makes deploying Python web applications easy and efficient. Whether you are working on a small Flask application or a large Django project, Gunicorn’s combination of simplicity, flexibility, and performance makes it a great choice for production environments.
By understanding its features, architecture, and best practices, you can maximize its potential for your web applications. Whether you are scaling to handle heavy traffic or optimizing your server to reduce latency, Gunicorn provides the tools and flexibility you need.