One of the fundamental concepts in Git is understanding the different Git stages and areas where changes can exist before they are committed to the repository. These areas serve as checkpoints, helping you control and manage what goes into your version history. In this article, we’ll dive into the primary Git areas and their roles in the development workflow.
Table of Contents
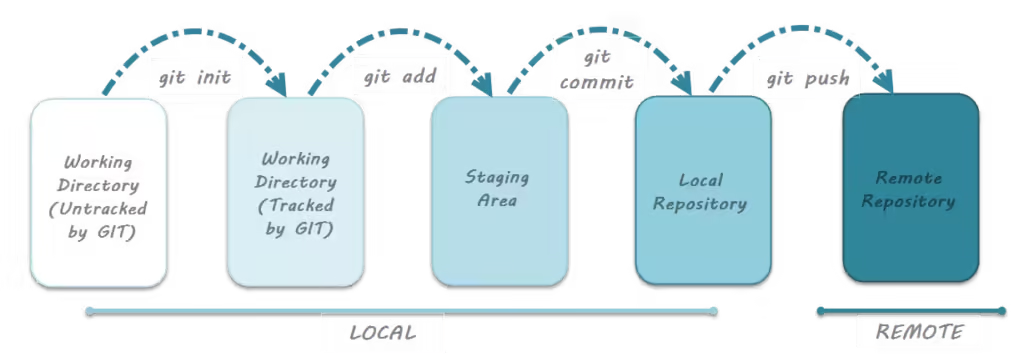
The Working Directory (Working Tree)
The working directory (sometimes called the working tree) is where you, the developer, do all your work. It’s the local folder on your machine where you create, modify, or delete files. When you clone a Git repository or check out a branch, Git copies the latest version of the files from the repository into the working directory.
At this stage, the files are in an “untracked” or “modified” state—Git is aware of the files, but it hasn’t yet marked which changes you intend to keep or commit. You can make changes freely here without affecting your version history.
What you do in the working directory:
- Create or delete files.
- Modify the content of existing files.
- Test and experiment with your code.
Key Point:
Changes in the working directory are not yet recorded in Git; they’re only local changes to your files.
The Staging Area (Index)
The staging area sometimes called the index, is a place where you can stage changes that are ready to be committed. Think of the staging area as a preparation step before committing to your version history. When you decide which changes to keep and commit, you move them from the working directory to the staging area using the git add command.
The staging area allows you to selectively prepare changes, which means you can choose to commit only specific files or parts of files, even if other parts are still in progress.
What you do in the staging area:
- Add changes using
git add <filename>.
Python- Stage individual changes in a file, if needed, using
git add -p.
Python- Unstage changes if you change your mind.
git reset <filename>
PythonKey Point:
The staging area is where you prepare the changes to be committed. It acts as a buffer between the working directory and the repository, giving you control over what gets committed.
The Repository (Commit History)
Once your changes are staged, you can commit them to the repository. The repository is the actual history of your project. A commit is a snapshot of your changes, saved with a unique hash ID, a commit message, and metadata (such as author and timestamp). Committing marks the changes as officially part of the project’s history.
After a commit, the staged changes are cleared from the staging area. Each commit is like a version of your project, and Git allows you to navigate through these commits to view or revert back to previous states of the code.
What you do in the repository:
- Commit changes using
git commit -m "Commit message"
Python- View history with git log or git history.
- Revert or reset changes using git revert or git reset.
Key Point:
The repository is where the project’s official history is stored. It’s the heart of version control, enabling you to track and manage all the changes made over time.
The Local Repository
The local repository refers to the .git directory within your project. It contains all the necessary files that Git uses to manage the version history, such as your commit history, branches, tags, and configuration settings.
When you perform operations like committing, Git stores this information locally within the .git directory. It’s hidden by default to prevent accidental modification.
Key Point:
The local repository contains the entire history of your project, and everything is stored in the .git directory.
The Remote Repository
The remote repository is an online or networked version of your repository, typically hosted on services like GitHub, GitLab, or Bitbucket. The remote repository serves as a centralized storage for your code, allowing multiple developers to collaborate on the same project.
When you push your commits, you send them from your local repository to the remote repository. Conversely, when you pull changes, you bring updates from the remote repository into your local copy.
What you do with the remote repository:
- Push changes using git push.
- Pull updates from the remote using git pull.
- Clone a repository to create a local copy with git clone.
Key Point:
The remote repository is where you store and share your code with collaborators. It ensures your project is backed up and available for others to access and contribute.
Switching Between Branches
When you use git checkout, Git switches your current working directory to match the state of the specified branch.
git checkout feature-branch
PythonThe contents of the working directory(i.e uncommited changes) are updated to reflect the files and folder structure of the branch you’re switching to.
branch 1>git checkout branch 2
PythonWhatever new, modified or deleted files are there in Branch 1 will move to Branch 2.
Example :
We are inside the feature branch, with the following changes
Feature Branch
create new file A
deleted file b
modifed file c
PythonNow we checkout to main branch, all those changes will move to the working directory of the main
Main Branch
create new file A
deleted file b
modifed file c
PythonWe again made a few changes in the Main branch
Main Branch
create new file A
deleted file b
modifed file c
added file x
deleted file y
create file z
PythonFinally, we checkout to Feature branch
Feature Branch
create new file A
deleted file b
modifed file c
added file x
deleted file y
create file z
PythonThis example shows how switching branches with git checkout changes the working directory to reflect the files and their content on the new branch.
What if, you don’t want to do so, The answer to this is git stash
Git Stash
Git Stash can be a lifesaver when you’re working on a feature or bug fix and suddenly need to switch contexts or work on a different task without committing your changes.
git stash # to stash
git stash list # list stashes
git stash apply # to apply stash
git stash pop # to remove stash
Git Workflow: How These Areas Fit Together
Here’s a simple workflow illustrating how these Git areas interact:
- Working Directory: You make changes to your files (e.g., edit index.html, create app.js).
- Staging Area: You stage the changes you want to keep (e.g., git add index.html).
- Repository: You commit the staged changes (e.g., git commit -m “Updated index.html”).
- Remote Repository: You push the commit to a remote repository (e.g., git push origin master).
This workflow allows you to carefully manage your changes, ensuring that only the changes you want to share are committed and pushed.
Conclusion
Git’s areas—the working directory, staging area, and repository—give developers full control over their project’s version history. By understanding and using these areas effectively, you can manage your codebase in a more organized and efficient manner. The local and remote repositories facilitate collaboration, enabling teams to work together seamlessly. Whether you’re a solo developer or part of a team, mastering these Git areas will enhance your workflow and improve version control practices.
1 thought on “Understanding Git stages: A Guide to Workflow Management”