Python, known for its simplicity, readability, and versatility, stands out as one of the most popular programming languages in the world today. Whether you’re working on data analysis, machine learning, or web development, Python provides an extensive toolkit to handle a wide array of tasks. Here’s a closer look at the major features that make Python an appealing choice for developers and hobbyists alike.
Table of Contents
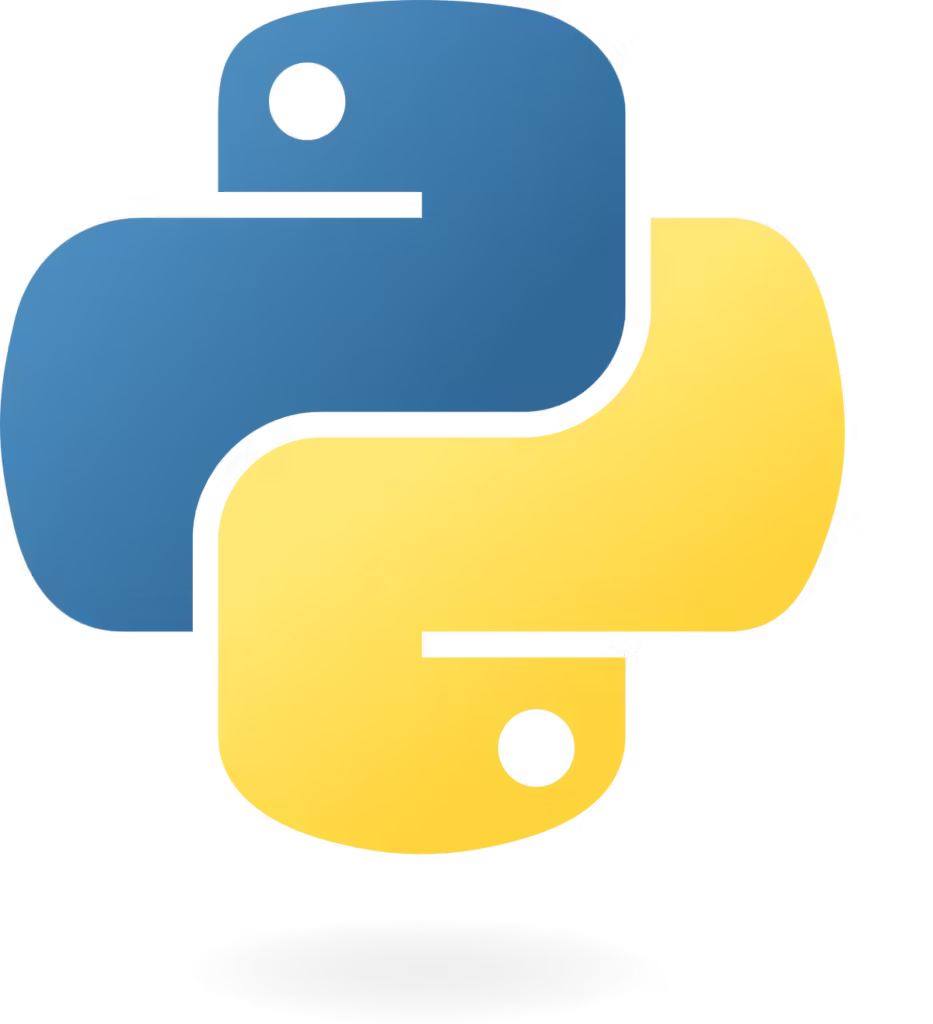
Simplicity and Readability
- Python’s easy-to-understand syntax promotes code readability, making it a favourite for both beginners and professionals. Its indentation-based structure enforces clean and consistent code.
- The minimalist approach allows for concise code, reducing the need for verbose instructions seen in languages like Java or C++.
High-Level Abstraction
- Python handles many low-level details like memory management and garbage collection. This allows developers to focus more on solving business problems rather than managing the nitty-gritty details of the system.
Dynamic Typing
- Variables in Python are dynamically typed, meaning you don’t need to declare their type upfront. Python will infer the type at runtime, which enhances flexibility and reduces boilerplate code.
pythonCopy codename = "John" # Python infers this as a string
age = 30 # Python infers this as an integer
Interpreted Language
- Python code is executed line by line by an interpreter, rather than compiled into machine code first. This makes testing and debugging more straightforward and reduces development cycles.
Comprehensive Standard Library
- One of Python’s greatest strengths is its vast standard library. Whether you need to handle file operations, communicate over the internet, or work with data structures, Python has built-in modules that can help.
- Popular modules include:
os
for operating system interactions.re
for regular expressions.http.client
for HTTP operations.
Object-Oriented Programming (OOP)
- Python’s robust support for OOP makes it easy to structure code in a modular, reusable way. It supports concepts like inheritance, polymorphism, and encapsulation, making complex applications more maintainable.
pythonCopy codeclass Car:
def __init__(self, model, year):
self.model = model
self.year = year
def drive(self):
print(f"The {self.model} is driving.")
my_car = Car("Toyota", 2020)
my_car.drive() # Outputs: The Toyota is driving.
Cross-Platform Compatibility
- Python runs on almost any operating system, from Windows to macOS to Linux, meaning code you write on one platform can run on others with minimal changes.
Supports Multiple Paradigms
- Python isn’t tied to a single programming paradigm. You can write in a procedural, object-oriented, or even functional programming style, thanks to built-in functions like
map()
,filter()
, and anonymous functions (lambdas).
Automatic Memory Management
- Python takes care of memory management automatically. Developers don’t need to manually allocate and deallocate memory, as Python uses a garbage collector to handle that behind the scenes.
Duck Typing and Dynamic Nature
- Python follows the principle of duck typing. If an object has the necessary methods, it doesn’t matter what type it is.
pythonCopy codeclass Bird:
def fly(self):
print("Flying")
class Plane:
def fly(self):
print("Taking off")
def take_off(entity):
entity.fly()
take_off(Bird()) # Outputs: Flying
take_off(Plane()) # Outputs: Taking off
Rich Ecosystem and Libraries
- Python’s ecosystem is vast. With over 400,000 packages on PyPI, you’ll find third-party libraries to help with almost any task—from NumPy for numerical operations to Flask for web development and TensorFlow for machine learning.
Concurrency and Parallelism
- Python supports multithreading and multiprocessing, allowing you to run parallel tasks and build efficient applications. Libraries like asyncio allow for asynchronous programming, ideal for I/O-bound operations.
Interactive and Scriptable
- Python’s interactive mode (REPL) allows you to quickly test code and see results in real-time. It’s also widely used for scripting, making it perfect for automating repetitive tasks.
Strong Community and Support
- Python boasts a large and vibrant community. From beginner-friendly tutorials to advanced topics discussed in conferences like PyCon, you can always find the support you need to grow as a Python developer.
AI, Data Science, and Beyond
- With libraries like Pandas, Matplotlib, and TensorFlow, Python has become the de facto language for data science, machine learning, and artificial intelligence. It’s now central to big data projects and research initiatives across industries.
Conclusion
Python’s comprehensive feature set, ease of use, and vast ecosystem make it a language that can handle almost any programming challenge. Whether you’re starting a new project or automating daily tasks, Python offers the tools and flexibility to accomplish your goals efficiently.