Flask is a lightweight, flexible, and powerful web framework for Python. It is designed to make getting started quick and easy, with the ability to scale up to complex applications. Flask is known for its simplicity, modularity, and fine-grained control.
Table of Contents
What is Flask?
Flask is a micro web framework written in Python. It is “micro” because it doesn’t come with pre-built tools like form validation or database abstraction layer — but you can plug them in as needed.
- Created by Armin Ronacher and released in 2010
- Based on WSGI toolkit and Jinja2 template engine
- Latest Version 3.1.0
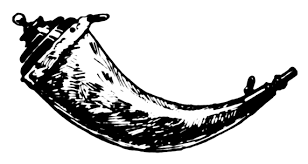
Installation
pip install flask
PythonCreate a file app.py
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello, Flask!"
PythonRun
flask run
PythonRouting
Define routes using @app.route():
@app.route('/user/<username>')
def show_user_profile(username):
return f'User: {username}'
PythonYou can also specify allowed types:
@app.route('/post/<int:post_id>')
def show_post(post_id):
return f'Post ID: {post_id}'
PythonTemplates (Jinja2)
Flask uses Jinja2 templating.
Example
from flask import render_template
@app.route('/')
def index():
return render_template('index.html', name="Alice")
Pythonindex.html
<h1>Hello {{ name }}!</h1>
PythonSyntax elements used in Jinja2
- {% … %} for Statements
- {{ … }} for Expressions to print to the template output
- {# … #} for Comments not included in the template output
- #… ## for Line Statements
{% … %} – Statements
Used for control structures like loops, conditionals, etc.
<ul>
{% for user in users %}
<li>{{ user }}</li>
{% endfor %}
</ul>
Python{{ … }} – Expressions (Output)
Used to print variables or expressions into the template output.
<h1>Welcome, {{ username }}!</h1>
<p>Uppercase Filter: {{ username | upper }}</p>
Python{# … #} – Comments
Used for non-rendered comments, completely removed from output.
{# This comment will not appear in the HTML source #}
Python#… ## for Line Statements
Line statements are used when you configure Jinja2 to use a simpler syntax, often in config files or scripts. They are not used by default in Flask templates unless you enable them.
# for user in users ##
- {{ user }}
# endfor ##
PythonRequest & Response
Request
from flask import request
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
return f"Welcome {username}"
PythonResponse
make_response() is a Flask function that allows you to create a Response object manually, so you can customize things like:
- Status code
- Headers
- Cookies
- MIME type (e.g., application/json, text/html)
Basic Example
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/')
def index():
response = make_response("Hello from Flask!")
response.status_code = 200 # Optional (default is 200)
response.headers["Custom-Header"] = "FlaskRocks"
return response
PythonExample: Returning JSON with Custom Status
from flask import Flask, jsonify, make_response
app = Flask(__name__)
@app.route('/custom-json')
def custom_json():
data = {"message": "This is a custom response"}
response = make_response(jsonify(data), 202)
response.headers['X-Special-Header'] = 'Yes'
return response
PythonStatic Files
- Flask serves static files from the static/ folder.
- Example URL: /static/style.css
Forms & Validation
Use WTForms with Flask-WTF:
pip install flask-wtf
Pythonfrom flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired
class NameForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
submit = SubmitField('Submit')
PythonDatabase Integration
With SQLite:
import sqlite3
conn = sqlite3.connect('database.db')
c = conn.cursor()
c.execute('''CREATE TABLE users (id INTEGER PRIMARY KEY, name TEXT)''')
conn.commit()
PythonWith SQLAlchemy:
pip install flask-sqlalchemy
Pythonfrom flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///site.db'
db = SQLAlchemy(app)
PythonBlueprints
- Blueprints in Flask are a way to organize your application into modular components. They allow you to split your app across multiple files, making it easier to maintain and scale.
- Think of a Blueprint as a “mini-app” — it can define its own routes, templates, static files, and more, and then be registered on the main app.
Why Use Blueprints?
Blueprints are essential for larger projects:
- Separation of concerns (e.g., auth logic, blog logic, admin panel, etc.)
- Easier team collaboration (developers can work on separate Blueprints)
- Reusability (you can create plug-and-play Blueprints)
- Cleaner code structure
Project Structure with Blueprints
Here’s a simple project layout:
myapp/
│
├── app.py
├── blog/
│ ├── __init__.py
│ └── routes.py
│
├── auth/
│ ├── __init__.py
│ └── routes.py
PythonCreating a Blueprint
Example: blog/routes.py
from flask import Blueprint, render_template
blog = Blueprint('blog', __name__, template_folder='templates')
@blog.route('/blog')
def blog_home():
return "Welcome to the Blog!"
PythonExample: auth/routes.py
from flask import Blueprint
auth = Blueprint('auth', __name__)
@auth.route('/login')
def login():
return "Login Page"
PythonRegistering Blueprints in app.py
from flask import Flask
from blog.routes import blog
from auth.routes import auth
app = Flask(__name__)
app.register_blueprint(blog, url_prefix='/')
app.register_blueprint(auth, url_prefix='/auth')
if __name__ == '__main__':
app.run(debug=True)
PythonThis will expose routes like:
- /blog → Blog homepage
- /auth/login → Auth login route
Blueprint Parameters
Blueprint supports several optional parameters:
- url_prefix: Add a common prefix to all routes in the Blueprint.
- template_folder: If templates are stored within the Blueprint folder.
- static_folder: If static files are stored locally for the Blueprint.
- static_url_path: Customize how static files are accessed via URL.
Blueprint('shop', __name__, url_prefix='/shop',
template_folder='templates', static_folder='static')
PythonFlask Extensions
Popular Flask extensions include:
- Flask-SQLAlchemy
- Flask-WTF
- Flask-Login
- Flask-Migrate
- Flask-Mail
- Flask-Caching
- Flask-CORS
Middleware
Middleware is a piece of code that runs before or after your main route handler is executed. It’s a great way to handle cross-cutting concerns such as:
- Logging
- Authentication
- Rate limiting
- Modifying requests or responses
- Error tracking
- Timing execution
- CORS handling (can also be done via extensions)
In Flask, middleware can be implemented using:
- Flask’s before_request and after_request hooks
- Custom WSGI middleware
- Flask Extensions (e.g., Flask-CORS, Flask-Limiter)
Using before_request and after_request
These decorators hook into the request lifecycle.
from flask import Flask, request
app = Flask(__name__)
@app.before_request
def log_request_info():
print(f"Request to: {request.path}")
print(f"Method: {request.method}")
@app.after_request
def add_custom_header(response):
response.headers["X-Custom-Header"] = "MyCustomValue"
return response
PythonCustom WSGI Middleware
Since Flask is built on WSGI, you can write pure WSGI middleware that wraps your entire application.
class SimpleMiddleware:
def __init__(self, app):
self.app = app
def __call__(self, environ, start_response):
print("WSGI Middleware: Before Request")
return self.app(environ, start_response)
app.wsgi_app = SimpleMiddleware(app.wsgi_app)
PythonUse this when you want to intercept the request at the lowest possible level—before Flask even processes it.
Middleware via Flask Extensions
Flask has a ton of middleware-like extensions that simplify adding features:
- Flask-CORS – Add CORS headers
- Flask-Limiter – Rate limiting
- Flask-HTTPAuth – Basic & token auth
- Flask-Login – Session-based authentication
- Flask-Compress – Gzip compression
When to Use Middleware
Middleware is a great choice when:
- The logic should apply to many routes.
- The logic is not specific to your business logic (like user login).
- You want to keep your route handlers clean and focused.
Error Handling
Flask provides built-in mechanisms to handle errors and define custom responses for various HTTP status codes. You can gracefully handle errors using decorators or middleware-like hooks.
Basic Error Handler Example
@app.errorhandler(404)
def page_not_found(e):
return render_template('404.html'), 404
PythonThis means: if a user visits a route that doesn’t exist, Flask will show 404.html instead of a plain 404 page.
Handling Multiple Error Types
@app.errorhandler(400)
def bad_request(error):
return "Bad Request!", 400
@app.errorhandler(500)
def internal_error(error):
return "Oops! Something broke on our end.", 500
PythonAuthentication & Authorization
Using Flask-Login:
pip install flask-login
PythonSetup login manager, user model, login views, etc.
from flask_login import LoginManager, UserMixin, login_user
login_manager = LoginManager(app)
class User(UserMixin):
...
PythonTesting Flask Apps
Use Python’s unittest or pytest.
import unittest
class FlaskTestCase(unittest.TestCase):
def test_index(self):
tester = app.test_client(self)
response = tester.get('/')
self.assertEqual(response.status_code, 200)
PythonDeployment
- Using Gunicorn (for production):
gunicorn app:app
PythonFlask-RESTful
Flask-RESTful is an extension for Flask that helps you quickly build REST APIs in a more structured and Pythonic way. It provides tools and abstractions to make it easier to handle things like:
- Routing
- Request parsing
- Input validation
- Output formatting
- Status codes
Why use Flask-RESTful?
Flask itself is very flexible, but that also means you have to do a lot manually. Flask-RESTful simplifies that by:
- Letting you create API resources as Python classes
- Handling common REST patterns (GET, POST, PUT, DELETE) out of the box
- Providing a cleaner way to work with HTTP responses and request data
Example
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'message': 'Hello, world!'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
PythonKey Concepts
- Resource: A class-based representation of your endpoint.
- Api: Wraps your Flask app and provides route management.
- reqparse: Helps you parse and validate request arguments.
Flask vs Flask-RESTful vs Flask-RESTX
Feature / Framework | Flask | Flask-RESTful | Flask-RESTX |
---|---|---|---|
Core Purpose | Micro web framework | Extension for building REST APIs | Enhanced REST API support & documentation |
API Structure | Function-based routes | Class-based resources (Resource ) | Same as RESTful, with namespaces |
Auto Docs (Swagger) | Not built-in | Not built-in | Swagger UI & OpenAPI support |
Request Parsing | Manual (request.args ) | reqparse | reqparse + better validation |
Validation | Manual | Basic (reqparse ) | Enhanced with models & fields |
Serialization | Manual (usually with jsonify ) | Basic (marshal ) | Improved marshal_with support |
Namespaces | No | No | Helps organize large APIs |
Extensibility | Very flexible | Good | Very good |
Learning Curve | Easiest | Easy | Slightly more to learn |
Best Use Case | Small apps, flexibility needed | Medium-sized REST APIs | Larger APIs, with built-in docs/validation |
Summary Recommendations:
- Use Flask: When you’re building small apps or want full control.
- Use Flask-RESTful: If you want cleaner API code but don’t need fancy docs or strict validation.
- Use Flask-RESTX: If you want an enterprise-ready API with Swagger docs, validation, and better organization.
KeyPoint
- Flask = Most basic, low-level control.
- Flask-RESTful = Cleaner REST APIs with class-based routes.
- Flask-RESTX = All of Flask-RESTful + Swagger UI + Namespaces + Better validation.
Advanced Topics
- Background jobs (Celery, APScheduler)
- WebSockets with Flask-SocketIO
- File upload handling
- JWT Authentication
- Flask Admin Panel
- Caching with Redis or Flask-Caching
Conclusion
Flask is a minimalist yet powerful tool for building web applications. Its flexibility, combined with Python’s simplicity, makes it a top choice for beginners and professionals alike.
Whether you’re building a blog or a microservice-based architecture, Flask gives you the tools and freedom to do it your way.