Python implementations provide a versatile approach to programming, known for their simplicity and readability. While CPython is the default and most popular choice, several alternative Python implementations cater to specific needs and environments. This article explores these options, highlighting their unique features and ideal use cases.
Table of Contents
CPython
Description: CPython is the standard implementation of Python, written in C. It serves as both an interpreter and a compiler for Python code. It’s the default and most widely used implementation of the Python programming language.
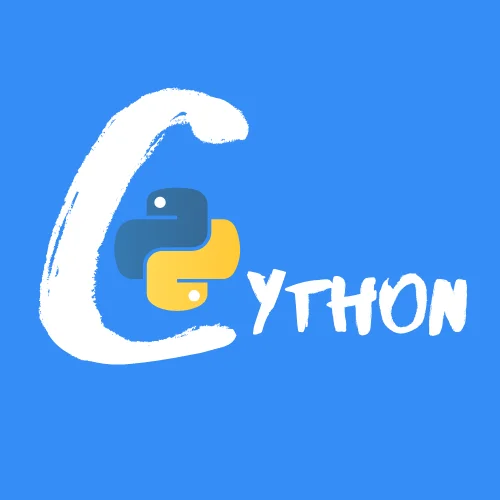
Working
- Compilation to Bytecode: When you run Python code using CPython, it first compiles the code into an intermediate bytecode. This bytecode is a lower-level, platform-independent representation of the source code.
- Execution by Python Virtual Machine (PVM): The CPython interpreter then executes this bytecode in the Python Virtual Machine (PVM), a part of CPython that interprets the bytecode line by line.
- Integration with C libraries: One key advantage of CPython is its ability to interact seamlessly with C-based libraries, making it fast for certain tasks, especially when writing performance-critical code using C extensions.
Features:
- Compiles Python code to bytecode for execution.
- Integrates seamlessly with C libraries.
- Supports a vast ecosystem of packages and libraries.
Use Cases:
General-purpose programming, web development, data analysis, and scientific computing.
PyPy
Description: PyPy is an alternative implementation focusing on performance and efficiency, featuring Just-In-Time (JIT) compilation.
Features:
- JIT compilation improves execution speed, especially for long-running applications.
- Reduced memory usage compared to CPython.
- Compatible with most Python libraries.
Use Cases:
Applications where speed is critical, such as web services, data processing, and scientific computations.
Jython
Description: Jython is an implementation of Python that runs on the Java platform, enabling integration with Java libraries.
Features:
- Translates Python code into Java bytecode.
- Offers seamless access to Java libraries and APIs.
- Allows Python developers to create Java applications.
Use Cases:
Building Java applications or working within a Java ecosystem, such as web applications using Java EE.
IronPython
Description: IronPython is designed for the .NET framework, enabling Python developers to leverage .NET libraries.
Features:
- Integrates with .NET languages and libraries.
- Allows for the development of Windows applications using Python.
- Supports dynamic typing and Python features.
Use Cases:
Developing applications in the Microsoft ecosystem, especially for Windows desktop applications or web applications using ASP.NET.
MicroPython
Description: MicroPython is a lightweight implementation of Python tailored for microcontrollers and embedded systems.
Features:
- Optimized for resource-constrained environments.
- Provides a subset of the Python standard library.
- Suitable for IoT devices and robotics.
Use Cases:
Embedded systems development, IoT applications, and educational projects with microcontrollers.
Stackless Python
Description: Stackless Python extends CPython with support for micro-threads or “tasklets,” focusing on concurrent programming.
Features:
- Enables lightweight, cooperative multitasking.
- Avoids the complexity of traditional threading.
- Facilitates the development of highly concurrent applications.
Use Cases:
Applications requiring high concurrency, such as network servers and real-time systems.
Brython
Description: Brython (Browser Python) is an implementation of Python that runs in the browser, translating Python code into JavaScript.
Features:
- Allows developers to write client-side web applications in Python.
- Provides a Pythonic way to interact with the Document Object Model (DOM).
- Reduces the need for JavaScript for web development.
Use Cases:
Front-end web development, especially for developers familiar with Python who want to create interactive web applications.
Nuitka
Description: Nuitka is a Python-to-C++ compiler that converts Python code into optimized C++ code, enhancing performance.
Features:
- Compiles Python code to native machine code.
- Produces standalone executables.
- Aims to improve execution speed while retaining Python semantics.
Use Cases:
Performance-critical applications, creating standalone applications, and situations where Python code needs to be packaged for distribution.
Why so many implementations?
The variety of Python implementations reflects the language’s adaptability and the ongoing evolution of software development. Each implementation addresses specific challenges, enhances performance, or facilitates integration with other technologies, ensuring that Python remains a versatile tool for developers across diverse domains. Here are some of the primary reasons:
- Performance Optimization: Different implementations focus on enhancing performance for specific scenarios:
- PyPy, for instance, utilizes Just-In-Time (JIT) compilation to speed up the execution of Python code, making it suitable for applications that require high performance over long periods.
- Nuitka compiles Python code to C++, improving execution speed and allowing the creation of standalone applications.
- Platform Compatibility: Some implementations are designed to operate within specific ecosystems or platforms:
- Jython enables integration with Java, allowing Python developers to use Java libraries and frameworks.
- IronPython serves the .NET environment, providing access to .NET libraries for Windows application development.
- Resource Constraints: In resource-constrained environments, such as embedded systems, lighter implementations are necessary:
- MicroPython is optimized for microcontrollers, enabling developers to write Python code for IoT devices and robotics without the overhead of full Python implementations.
- Concurrency and Multithreading: Different implementations address concurrency in unique ways:
- Stackless Python supports micro-threads, simplifying concurrent programming and making it easier to handle high levels of concurrency without traditional threading complexities.
- Web Development: Some implementations cater to web development needs:
- Brython translates Python code to JavaScript for client-side web development, allowing Python developers to create interactive web applications without needing to switch to JavaScript.
Why Python is not directly implemented in assembly language?
- While it is theoretically possible to implement Python directly in assembly language, the practical challenges and limitations associated with such an approach make it unfeasible.
- Instead, using a high-level language like C allows for greater portability, easier development and maintenance, and the ability to leverage existing libraries and tools, making Python a powerful and versatile programming language.
Can we use C on Cpython?
Yes, you can use C in Python, and there are several ways to integrate C code with Python. Here are some common methods to leverage C functionality within your Python applications:
- Python C API: You can write C extensions for Python using the Python C API. This allows you to create shared libraries that can be imported directly into Python.
- ctypes: The ctypes module in Python allows you to call functions in DLLs or shared libraries directly from Python. Useful for calling existing C libraries without needing to write a separate extension module.
- cffi (C Foreign Function Interface): cffi is a Foreign Function Interface for Python that allows calling C code from Python easily and provides more features compared to ctypes.
- SWIG (Simplified Wrapper and Interface Generator): WIG is a tool that connects C/C++ code with various high-level programming languages, including Python. It generates wrapper code to facilitate this integration.
- Boost.Python: A part of the Boost C++ Libraries, Boost. Python is specifically designed to help create Python bindings for C++ code.
How to check my Python implementation?
import platform
print(platform.python_implementation()) # Shows the Python implementation
print(platform.python_version()) # Shows the Python version
Output:
CPython
3.9.2
Conclusion
Python’s versatility is not limited to a single implementation. Each alternative implementation offers unique features and advantages that cater to specific use cases and environments. By understanding these implementations, developers can choose the best option for their projects, whether they prioritize speed, compatibility, or resource efficiency.