Concurrency is a crucial concept in programming, especially when dealing with tasks that need to be executed simultaneously or when optimizing for time.
Python offers multiple ways to handle concurrency, with two of the most popular approaches being asyncio
and threads. While both serve the purpose of making applications more efficient, they have distinct mechanisms, use cases, and advantages. This article explores the differences between asyncio
and threads, helping you choose the right tool for your next project.
Table of Contents
What is asyncio?
asyncio is Python’s built-in library designed for asynchronous programming. It enables writing single-threaded concurrent code using the async and await keywords. Unlike traditional threading, where multiple threads execute simultaneously, asyncio operates on an event loop model, meaning tasks cooperate by yielding control, allowing other tasks to run.
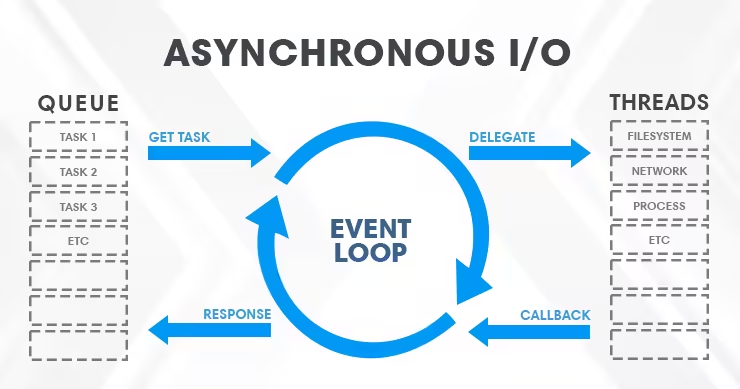
How it Works:
asyncio
primarily works on a single thread. It is built around the concept of an event loop, where tasks are executed concurrently but not in parallel. Unlike multithreading or multiprocessing, which involve running tasks on separate threads or CPU cores, asyncio
handles multiple tasks by allowing them to run cooperatively within the same thread. This means tasks “take turns” executing, yielding control back to the event loop when they are waiting for an operation to complete (like network requests or file I/O).
- Single-threaded: The core idea of
asyncio
is that you don’t need multiple threads or processes to handle concurrency. All tasks run on a single thread, but they are non-blocking, meaning tasks yield control when they’re waiting for something (like a response from a web server). - Cooperative multitasking: Each task explicitly yields control using
await
. The event loop then checks if other tasks are ready to run, creating an illusion of concurrency while still only using one thread.
import asyncio
async def task1():
print("Task 1 started")
await asyncio.sleep(2)
print("Task 1 finished")
async def task2():
print("Task 2 started")
await asyncio.sleep(1)
print("Task 2 finished")
async def main():
await asyncio.gather(task1(), task2())
asyncio.run(main())
PythonHere, both task1 and task2 run on the same thread, but asyncio switches between them as they await (i.e., during the await asyncio.sleep() calls). The tasks run concurrently (appearing to run at the same time), even though they are not actually running in parallel.
Key Features of asyncio:
- Event Loop: The core of asyncio is its event loop. Tasks can register themselves on this loop, which schedules and runs them asynchronously.
- Non-blocking I/O: One of asyncio’s main advantages is its ability to handle non-blocking I/O efficiently. It’s perfect for operations like network requests, file handling, or database queries, where tasks spend a lot of time waiting for responses.
- Lightweight: Since asyncio doesn’t rely on multiple threads or processes, it requires less memory overhead, making it scalable for applications that handle thousands of concurrent connections, such as web servers.
- Concurrency, not parallelism: asyncio provides concurrency by letting the program switch between tasks while waiting for I/O or other blocking operations, but it does not run tasks in parallel.
- Simpler code structure: The async and await syntax makes writing asynchronous code straightforward to use, resembling regular synchronous code in many ways.
Best Use Cases for asyncio:
- Network Applications: Applications that handle many connections at once, such as web servers or network tools, benefit greatly from asyncio.
- Web Scraping: When scraping multiple websites concurrently, asyncio can be more efficient than threading.
- File I/O Operations: Tasks involving reading from or writing to files are perfect for asyncio since they often spend time waiting for the disk.
What are Threads?
Threading is a well-known technique for concurrency in Python, enabling multiple tasks to run simultaneously. Threads are separate flows of execution that share the same memory space but execute independently. Python’s threading module makes it easy to launch new threads in an application.
Key Features of Threads:
- True Parallelism: In theory, threads offer the ability to execute tasks in parallel on different CPU cores. However, Python’s Global Interpreter Lock (GIL) can limit this when using CPython, meaning that while multiple threads can exist, they don’t always execute in parallel, particularly for CPU-bound tasks.
- Thread Management: Threading allows developers to write multithreaded applications that perform multiple tasks at once. However, managing threads can be complex, especially when it comes to synchronizing access to shared resources or avoiding race conditions.
- Suitable for CPU-bound Tasks: Threads shine when tasks involve significant computation. Even though Python’s GIL prevents true parallelism for many CPU-bound tasks, the ability to offload some work to different threads can still provide performance benefits.
Best Use Cases for Threads:
- CPU-bound Tasks: If your task involves heavy computation that can be split across multiple cores, threads (or even better, multiprocessing) may be the right choice.
- Blocking I/O: Threads are still effective for I/O-bound tasks, especially when asynchronous programming might add unnecessary complexity.
- Background Processes: For running background operations, such as logging, that don’t need constant monitoring, threading can be a simple solution.
Key Differences: asyncio vs Threads
Feature | asyncio | Threads |
---|---|---|
Concurrency Model | Cooperative multitasking (tasks voluntarily yield control) | Preemptive multitasking (OS manages task switching) |
Parallel Execution | No true parallelism (single-threaded) | Allows parallel execution (with limitations) |
Overhead | Lightweight, low memory usage | Higher memory overhead, especially with many threads |
Code Complexity | Simpler with async /await keywords | More complex due to thread management and potential race conditions |
Best for | I/O-bound tasks (networking, file I/O) | CPU-bound tasks, blocking I/O, background operations |
Choosing Between Asyncio and Threads
The choice between asyncio
and threading largely depends on the nature of your task. If you are dealing with I/O-bound operations, such as making web requests, reading files, or querying databases, asyncio
is likely the more efficient choice. Its non-blocking nature allows for handling many tasks concurrently with lower memory overhead, which makes it suitable for tasks that require waiting for external events.
On the other hand, if you need to run CPU-bound tasks, threading might be more appropriate, despite Python’s GIL limitations. Threads allow for parallelism to some extent and are more suited for scenarios where tasks need to perform computational work in the background while others continue executing.
A Real-World Example
Suppose you’re building a web server that handles thousands of connections per second. Since the server spends much of its time waiting for responses from databases or external services, using asyncio
would be the optimal choice. It can manage the many simultaneous connections efficiently without needing the overhead of multiple threads or processes.
Now consider a background image processing task in which you apply filters or transformations to large images. In this case, threading (or even multiprocessing) might be better because the tasks are CPU-intensive and don’t involve much waiting.
Is it Python-specific?
The concept behind asyncio, which is asynchronous programming based on an event loop, is not Python-specific. It is a general concurrency model found in many programming languages and frameworks. While Python’s asyncio library is its own implementation, the underlying concepts of non-blocking I/O, event-driven programming, and cooperative multitasking exist in various forms across multiple programming ecosystems.
Similar Concepts in Other Languages:
Language | Concurrency Model |
---|---|
JavaScript | Single-threaded, event-driven (Node.js),similar to Python asyncio |
Go | Lightweight concurrency with goroutines |
C# (.NET) | Task-based asynchronous patterns using async/await, similar to Python asyncio |
Rust | Single-threaded, event-driven (Node.js), similar to Python asyncio |
Java | Asynchronous tasks with CompletableFutures and ExecutorService |
While the idea of asynchronous tasks is universal, the specific concurrency model can differ. For example, Goroutines in Go are much lighter than Python threads and can run in parallel on multiple cores, while Python’s asyncio
sticks to cooperative multitasking on a single thread.
Conclusion
Both asyncio
and threads offer powerful mechanisms for handling concurrency, but they are optimized for different use cases. asyncio
is best suited for I/O-bound tasks where non-blocking operations can maximize efficiency, while threading is better for CPU-bound tasks or scenarios where you need true parallelism. Understanding the strengths and limitations of each will help you choose the right tool for your application, ultimately leading to better performance and resource management.
5 thoughts on “Understanding Asyncio and Threads in Python”