In computing, the concepts of synchronous and asynchronous execution play crucial roles in how tasks are managed and processes are carried out. While both approaches have merits, they are suitable for different use cases. Understanding the key differences between synchronous and asynchronous execution helps design more efficient and responsive applications.
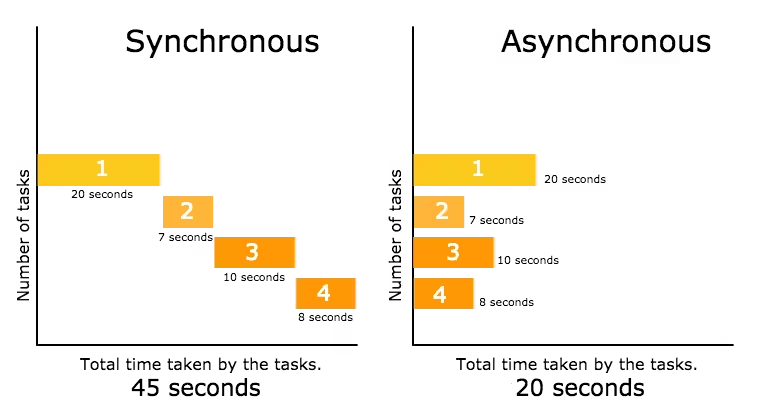
Table of Contents
Synchronous Execution
Definition: Synchronous execution refers to the process where tasks are executed one after the other, in a sequence. Each task must be completed before the next one begins, which ensures that tasks are processed in a predictable and orderly manner.
Single Threaded:

Once Task 1 is executed, then task 2 will start soon.
Multi-Threaded:

- As we have three threads, each thread picks one task.
- Task 4 will be executed by any of the free threads.
This design leads to inefficient resource utilization.
Although multiple threads are available, only one is active at a time because the tasks are executed synchronously. As a result:
- Thread-2 remains idle while Thread-1 completes Task-1.
- Thread-3 remains idle until both Task-1 and Task-2 are finished.
This defeats the purpose of using multiple threads. If the tasks are independent, Threads 2 and 3 should be able to start their respective tasks immediately, rather than waiting. Proper concurrency would allow all threads to be utilized effectively, improving performance and reducing idle time.
Characteristics of Synchronous Execution:
- Sequential Task Flow: Each task waits for the previous task to complete before it starts.
- Blocking Nature: When a task is in progress, other tasks are blocked from execution until it finishes.
- Simpler to Implement: Writing synchronous code is straightforward since you can follow a linear, step-by-step approach to task execution.
- Predictable Behavior: Since tasks are executed one at a time, it is easier to predict the flow and manage the outcomes of different operations.
Example of Synchronous Execution:
def task_a():
print("Task A completed")
def task_b():
print("Task B completed")
task_a() # Task A must finish before Task B starts
task_b()
PythonIn this example, task_b() will not execute until task_a() finishes its execution.
When to Use Synchronous Execution:
- When Order is Important: Some applications require a strict sequence of operations, such as banking transactions or writing to a database.
- For Simpler Applications: In smaller applications where performance and responsiveness aren’t a major concern, synchronous execution may suffice.
- When Tasks Depend on Each Other: If task B requires the result of task A, synchronous execution ensures that task A finishes first.
Asynchronous Execution
Definition: Asynchronous execution allows multiple tasks to run independently of each other. Tasks can start, pause, or complete without waiting for others to finish, which can lead to more efficient resource utilization and faster response times, especially in I/O-heavy operations.
Characteristics of Asynchronous Execution:
- Non-blocking Nature: A task does not block other tasks from being executed. While waiting for a task to complete (e.g., a network request), other tasks can run.
- Concurrency: Multiple tasks can be in progress at the same time, improving the overall performance, especially in applications that involve network requests, file I/O, or database queries.
- More Complex to Implement: Asynchronous programming often requires managing callbacks, promises, or event loops, which can introduce complexity.
- Better Scalability: Asynchronous execution is well-suited for handling a large number of concurrent tasks, such as in web servers or real-time applications.
Single Thread

Multiple Thread

Example of Asynchronous Execution:
import asyncio
async def task_a():
print("Task A completed")
async def task_b():
print("Task B completed")
async def main():
await asyncio.gather(task_a(), task_b()) # Tasks A and B can run concurrently
asyncio.run(main())
PythonHere, task_a() and task_b() run concurrently without blocking each other, allowing both tasks to finish more quickly.
Does task_a and task_b run on different CPUs.
It does not run tasks on different CPUs.
- It uses Python’s asyncio module to run tasks concurrently, but on a single thread and CPU core.
- The concurrency is cooperative, meaning tasks yield control using await, and the event loop switches between them.
If You Want to Use Multiple CPUs
You’d need to use the multiprocessing module instead of asyncio.
When to Use Asynchronous Execution:
- I/O-bound Applications: Asynchronous execution is ideal for applications that spend a lot of time waiting on external resources, such as web servers, file systems, or databases.
- High Responsiveness: In UI-based applications or real-time systems, asynchronous programming ensures that the application remains responsive to user input while background tasks run.
- Parallel Processing: Asynchronous execution allows multiple independent tasks to progress without waiting for one to finish, which is useful in modern web applications and services.
Key Differences
Aspect | Synchronous | Asynchronous |
---|---|---|
Execution Flow | Tasks run sequentially | Tasks can run concurrently |
Task Waiting | A task must be completed before the next one starts | Tasks do not wait for others to finish |
Blocking | Blocking | Non-blocking |
Complexity | Easier to implement | More complex (callbacks, promises, async/await) |
Use Cases | Tasks that must run in sequence | Tasks involving I/O, network, or background processes |
Performance | Less efficient for I/O operations | Highly efficient for I/O-heavy operations |
Scalability | Less scalable in handling concurrency | Highly scalable, ideal for real-time applications |
Real-World Examples
- Synchronous: File Processing: Imagine processing a large file. In synchronous execution, the program reads and processes each line one after the other. If the file is large, this will block the system from doing other tasks until the file processing completes.
- Asynchronous: Web Servers
- In web applications, asynchronous execution is common. For example, when a user makes a request to a server, the server can handle multiple requests at once without waiting for one request to finish. This allows for faster response times and better scalability, crucial for services like APIs or real-time applications.
Analogies
Synchronous: You are in a queue to get a movie ticket. You cannot get one until everybody in front of you gets one, and the same applies to the people queued behind you.
Asynchronous: You are in a restaurant with many other people. You order your food. Other people can also order their food, they don’t have to wait for your food to be cooked and served to you before they can order. In the kitchen, restaurant workers are continuously cooking, serving, and taking orders. People will get their food served as soon as it is cooked.
Choosing Between Synchronous and Asynchronous
- When designing an application, the choice between synchronous and asynchronous execution depends on the nature of the tasks at hand. If you’re working on a system where tasks rely on each other and must follow a strict order, synchronous execution is preferable. However, if your application needs to handle many independent tasks simultaneously or perform long-running operations without freezing, asynchronous execution will yield better results.
Ultimately, modern applications often benefit from a combination of both, where critical sections of code run synchronously and non-critical, long-running operations run asynchronously in the background.
Conclusion
Synchronous and asynchronous executions are foundational concepts in programming, each with distinct advantages and use cases. By understanding their differences, developers can choose the right approach for their specific needs, leading to more efficient, scalable, and responsive applications.
3 thoughts on “Synchronous vs Asynchronous: Key Differences Explained”