Python programmers should be familiar with four fundamental data structures: lists, dictionaries, tuples and sets. Each of these structures has unique properties and use cases. In this article, we’ll delve into the details of these data structures, covering their creation, usage, and common operations.
Table of Contents
Let’s start the fun
Guess the data structures best suited
Question
- Tracking Unique Product SKUs in a Store Inventory.
- Storing Customer Information with Phone Numbers.
- Storing Coordinates of Locations (e.g., Latitude, Longitude)
- Managing Items in a Shopping Cart
- Storing the List of Students in a Classroom
- Storing the Results of a Survey (e.g., Answers to Multiple-Choice Questions)
- Storing the Sequence of Events in a Log File (e.g., User Actions or Errors)
- Storing the Grades of Students in a Class (with Names and Grades)
- Removing Duplicate Emails from a Mailing List
- Storing Date and Time Information for Logging Events (Immutable)
- Storing Items in a Playlist (with Ability to Reorder)
- Checking for Common Items Between Two Lists (e.g., Common Products in Two Stores)
- Storing Configuration Settings for an Application
- Tracking User Activity (Immutable, Fixed Data)
- Sorting and Filtering a List of Products by Price
- You have collections of books in two different libraries. You want to know all the unique books you have.
- You want to know which employees are both working in the company and eligible for the health benefit.
- You want to find out which students have not completed their assignments.
Answer
- The Set. Set automatically ensures that all items are unique, which is ideal for tracking product SKUs, as you do not want duplicates. Additionally, checking if an SKU is already in the inventory is efficient with sets.
- The Dictionary. Dictionary allows you to store data as key-value pairs, where the key is the customer’s name and the value is their phone number. Dictionaries provide fast lookups by key, making retrieving phone numbers based on customer names easy.
- The Tuple. The Tuple is immutable, meaning the data cannot be changed once created. This is perfect for storing fixed data like coordinates where you do not want any accidental modifications.
- The List. The list maintains the order of elements, and you can easily add, remove, or reorder items. This makes them perfect for tasks like managing a shopping cart where the user may change the contents dynamically.
- The Set. Since sets automatically handle uniqueness and we don’t care about the order of students, it is ideal for this use case. We can easily check whether a student is already in the classroom.
- The Dictionary. It allows you to store the question as the key and the response as the value, making it easy to access and manipulate the responses.
- The List. Lists maintain the order of elements, which is critical for logging events, and you can easily append new events to the list.
- The Dictionary. The dictionary allows you to store student names as keys and their corresponding grades as values, providing an efficient way to retrieve grades based on the student’s name.
- The Set. Sets automatically remove duplicates, so you can easily add all emails to a set and ensure there are no duplicates left.
- The Tuple. Since tuples are immutable, they can be used to store fixed data like date and time, ensuring that the timestamp remains unchanged.
- The List. Lists allow you to maintain the order of items and provide flexibility to reorder or modify the playlist as needed.
- The Set. Sets allow for fast membership checking and can easily be used to find the intersection of two sets, helping you efficiently identify common items between them.
- The Dictionary. It allows you to store the configuration settings as key-value pairs, making it easy to retrieve and modify individual settings.
- The Tuple. Since tuples are immutable, they are well-suited for storing fixed, unchanging information like user activity logs.
- The List. Lists allow for ordered data, making it easy to sort products by price. You can also filter the list by applying certain conditions (e.g., products with a price above a certain threshold).
- Set. You can perform a set union operation to get the unique book.
- The Set. The intersection operation helps you identify the employees that meet both criteria.
- The Set. You can use the Set Difference operation.
If you can answer all the questions, you may skip this article.
Python Collections
There are four collection data types in the Python programming language:
- A dictionary is a collection that is ordered** and changeable. No duplicate members.
- A list is a collection that is ordered and changeable. Allows duplicate members.
- Tuple is a collection that is ordered and unchangeable. Allows duplicate members.
- Set is a collection that is unordered, unchangeable*, and unindexed. No duplicate members.
Properties | Dictionary | List | Set | Tuple |
Duplicate | No | Yes | No | Yes |
Mutable | Yes | Yes | Yes | No |
Ordered | Yes | Yes | No | Yes |
Indexed | Yes | Yes | No | Yes |
Symbol | {} | [] | {} | () |
Example | {‘key1’:”value”} | [1,2,3] | {1,2,3} | (1,2,3) |
Definition | Key-Value Pairs | Ordered Collection | Unique Collection | Immutable List |
Note:
- Sets are mutable (can add or remove elements only), but we can’t change the elements of a set
- Tuples are non-mutable, we cannot change, add or remove items after the tuple has been created.
- Dictionaries are indexed by keys, while lists and tuples are indexed by a range of numbers
- Dict[‘name’]
- mylist[0] or mytuple[2]
- As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered.
- Ordered means that the elements of the collection have a specific order. In this article by order means insertion order
To Remember
- A list is like a todo list with a specific order;
- A tuple is like a list, where one can’t change items in the list
- Set where duplicates are not allowed
- A dictionary is simply Key-Value Pairs
Data Structure | Access Time | Search Time | Insertion Time | Deletion Time |
---|---|---|---|---|
List | O(1) | O(n) | 1. O(1): append 2. O(n): insert at the position due to shifting elements | 1. O(n): remove by value 2. O(n): remove by index as shifting elements to fill the gap 3.O(1): remove from end. Shifting not required |
Set | O(1) | O(1) | O(1) | O(1) |
Tuple | O(1) | O(n) | Not applicable | Not applicable |
Dictionary | O(1) | O(1) | O(1) | O(1) |
Note
- In sets, search time is O(1) on average because they are implemented using a hash table.
Dictionary
- Collection of key-value pairs
- Duplicates are not allowed
- Ordered
- Indexed based on keys
- {}
As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered.
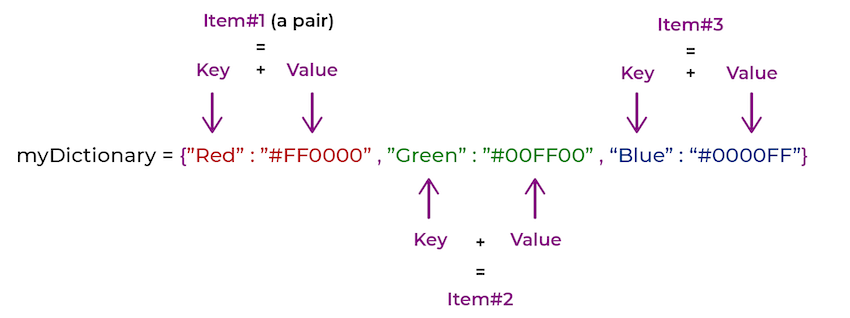
Accessing Dictionary Elements
# Example of a dictionary
my_dict = {"name": "Alice", "age": 25, "city": "New York","email":"alice@example.com"}
# Accessing the value associated with the key 'name'
print(my_dict["name"]) # Output: Alice
PythonModifying a Dictionary
# Changing the value of the key 'age'
my_dict["age"] = 26
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York'}
PythonAdding and Removing Key-Value Pairs
# Adding a new key-value pair
my_dict["email"] = "alice@example.com"
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'city': 'New York', 'email': 'alice@example.com'}
# Removing a key-value pair
del my_dict["city"]
print(my_dict) # Output: {'name': 'Alice', 'age': 26, 'email': 'alice@example.com'}
PythonDictionary Methods
keys = my_dict.keys()
print(keys) # Output: dict_keys(['name', 'age', 'email'])
values = my_dict.values()
print(values) # Output: dict_values(['Alice', 26, 'alice@example.com'])
items = my_dict.items()
print(items) # Output: dict_items([('name', 'Alice'), ('age', 26), ('email', 'alice@example.com')])
age = my_dict.get("age")
print(age) # Output: 26
age = my_dict["age"]
print(age) # Output: 26
city = my_dict.get("city", "Unknown")
print(city) # Output: Unknown
email = my_dict.pop("email")
print(email) # Output: alice@example.com
print(my_dict) # Output: {'name': 'Alice', 'age': 26}
Pythonmy_dict.get(“age”)
- Behavior: If the key “age” exists in the dictionary, it returns its value. If the key does not exist, it returns None (or a default value you specify).
- No KeyError: This approach is safer because it won’t raise a KeyError if the key is missing.
- Use get when the key might not exist and when you want a fallback or default value without raising an error.
- Custom Default: You can specify a default value as the second argument:
age = my_dict.get("age", 0) # Returns 0 if "age" key is not found.
Pythonmy_dict[“age”]
- Behavior: If the key “age” exists, it returns its value. If the key does not exist, it raises a KeyError.
- Use this when you’re confident the key exists in the dictionary and its absence is considered an error in the context.
- Error Handling: You must ensure the key is present in the dictionary, or use a try-except block to handle potential errors:
try:
age = my_dict["age"]
except KeyError:
age = 0
PythonIteration
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
#By default, iterating over a dictionary only gives you the keys:
for key in my_dict: # This is equivalent to my_dict.keys():
print(key)
'''
name
age
city
'''
#getting value by key
for key in my_dict:
print(my_dict[key])
'''
Alice
25
New York
'''
#If you want to explicitly access the keys, you can also use the .keys() method:
for key in my_dict.keys():
print(key)
'''
name
age
city
'''
for value in my_dict.values():
print(value)
'''
Alice
25
New York
'''
for key, value in my_dict.items():
print(f"{key}: {value}")
'''
name: Alice
age: 25
city: New York
'''
# enumerate With keys
for index, key in enumerate(my_dict.keys()):
print(f"Index {index}: Key {key}")
'''
Index 0: Key name
Index 1: Key age
Index 2: Key city
'''
# enumerate With values
for index, value in enumerate(my_dict.values()):
print(f"Index {index}: Value {value}")
'''
Index 0: Value Alice
Index 1: Value 25
Index 2: Value New York
'''
PythonLists
- Ordered collection of items
- Mutable
- Allow Duplicates
- Indexed
- []
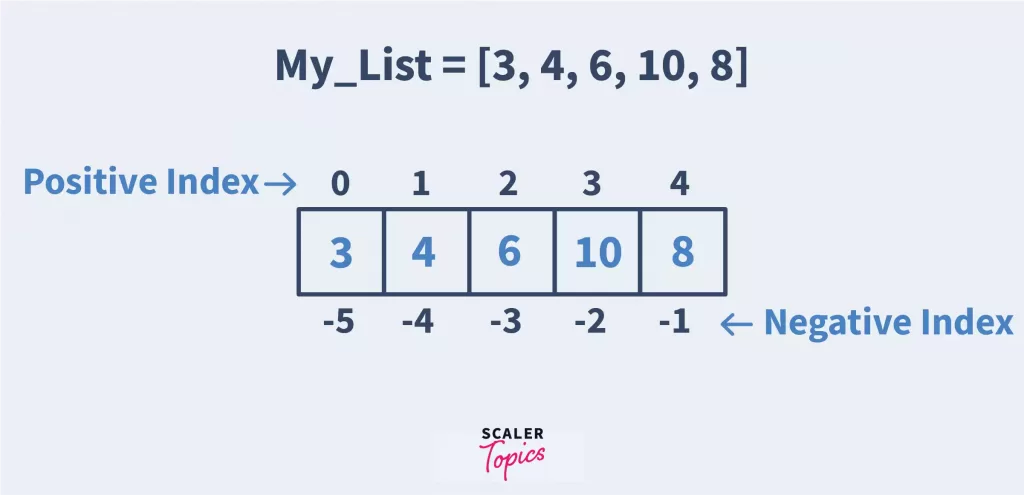
Accessing List Elements
# Example of a list
my_list = [1, 2, 3, "Python", 4.5]
# Accessing the first element
print(my_list[0]) # Output: 1
# Accessing the fourth element
print(my_list[3]) # Output: Python
PythonModifying a List
# Changing the second element
my_list[1] = "Changed"
print(my_list) # Output: [1, 'Changed', 30, 'Python', 4.5]
PythonList Methods
my_list.append(6)
print(my_list) # Output: [1, 'Changed', 30, 'Python', 4.5, 6]
my_list.insert(2, "Insert")
print(my_list) # Output: [1, 'Changed', 'Insert', 30, 'Python', 4.5, 6]
my_list.remove(3)
print(my_list) # Output: [1, 'Changed', 'Insert', 'Python', 4.5, 6]
element = my_list.pop(1)
print(element) # Output: Changed
print(my_list) # Output: [1, 'Insert', 'Python', 4.5, 6]
numbers = [4, 2, 9, 1]
numbers.sort()
print(numbers) # Output: [1, 2, 4, 9]
numbers.reverse()
print(numbers) # Output: [9, 4, 2, 1]
PythonIteration
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
for i in range(len(my_list)):
print(f"Index {i}: {my_list[i]}")
for index, item in enumerate(my_list):
print(f"Index {index}: {item}")
'''
1
2
3
4
5
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
'''
PythonSet
- Unordered
- Duplicates not allowed
- Indexing not available
- Mutable
- {}
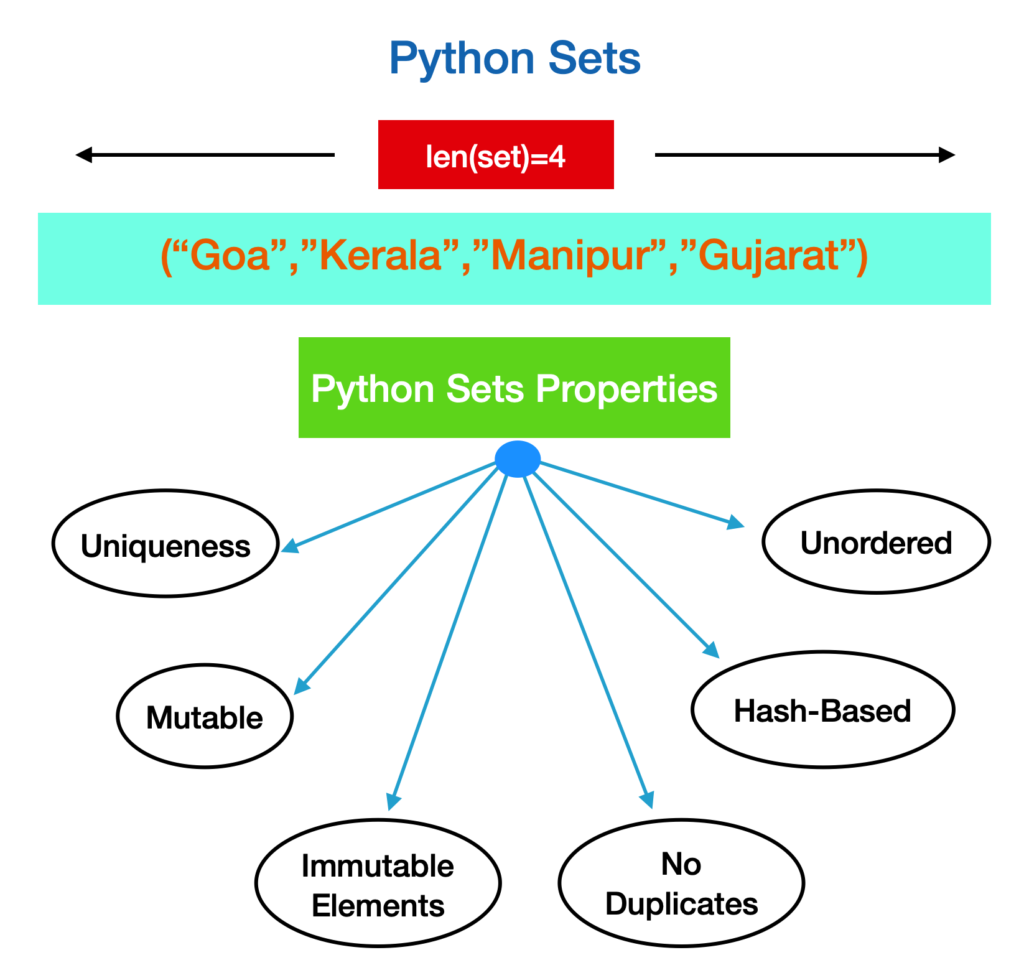
Creating a Set
# Example of a set
my_set = {1, 2, 3, 4, 5}
# Another way to create a set
another_set = set([1, 2, 3, 4, 5])
PythonAdding and Removing Items
# Adding an item
my_set.add(6)
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
# Removing an item
my_set.remove(2)
print(my_set) # Output: {1, 3, 4, 5, 6}
# Removing an item that may or may not
PythonWe can only add or remove elements, and can’t modify them
Set Methods
copy_set = my_set.copy()
print(copy_set) # Output: {1, 3, 4, 5, 6}
my_set.clear()
print(my_set) # Output: set()
my_set = {1, 2, 3}
element = my_set.pop()
print(element) # Output: 1 (or 2 or 3, depending on the internal order)
print(my_set) # Output: {2, 3} (or {1, 3} or {1, 2})
my_set = {3, 1, 4, 2}
sorted_list = sorted(my_set) # This will return {1, 2, 3, 4}
PythonIterate
my_set = {1, 2, 3, 4, 5}
for item in my_set:
print(item)
for index, item in enumerate(my_set):
print(f"Index {index}: {item}")
'''
1
2
3
4
5
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
'''
PythonIndexing is not available, so we can iterate using an index like in the case of a list or tuple
Tuples
- Ordered
- Immutable
- Duplicates allowed
- Indexed by number
- ()
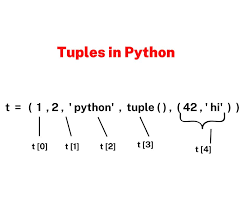
Accessing Tuple Elements
# Example of a tuple
my_tuple = (1, 2, 3, "Python", 4.5)
# Creating a tuple with a single element
single_element_tuple = (5,)
# Accessing the first element
print(my_tuple[0]) # Output: 1
# Accessing the last element
print(my_tuple[-1]) # Output: 4.5
PythonTuple Unpacking
# Unpacking a tuple
a, b, c, d, e = my_tuple
print(a) # Output: 1
print(d) # Output: Python
PythonImmutability of Tuples
# Trying to change an element will result in an error
# my_tuple[1] = "Changed" # This will raise a TypeError
# However, you can concatenate tuples to create a new one
new_tuple = my_tuple + ("New Element",)
print(new_tuple) # Output: (1, 2, 3, 'Python', 4.5, 'New Element')
PythonTuple Methods
count = my_tuple.count(2)
print(count) # Output: 1
index = my_tuple.index("Python")
print(index) # Output: 3
PythonTuple Packing and Unpacking
Tuple packing refers to the process of assigning multiple values to a single tuple, while tuple unpacking is the reverse operation.
# Packing a tuple
packed_tuple = 1, 2, "Python"
# Unpacking a tuple
x, y, z = packed_tuple
print(x) # Output: 1
print(z) # Output: Python
PythonIterate
my_tuple = (1, 2, 3, 4, 5)
for item in my_tuple:
print(item)
for i in range(len(my_tuple)):
print(f"Index {i}: {my_tuple[i]}")
for index, item in enumerate(my_tuple):
print(f"Index {index}: {item}")
'''
1
2
3
4
5
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
Index 0: 1
Index 1: 2
Index 2: 3
Index 3: 4
Index 4: 5
'''
PythonTuples vs. Lists
While both tuples and lists are used to store collections of items, the choice often depends on whether the collection needs to be mutable or immutable.
- Mutability: Lists are mutable (modifiable), while tuples are immutable.
- Performance: Tuples can be more memory-efficient and slightly faster than lists, especially when dealing with large data collections that do not need modification.
- Usage: Use tuples when you want to ensure that the data remains constant, such as when the structure of data is integral to the program (e.g., coordinates, RGB values).
Tuples as keys in dictionaries (since they are hashable)
Tuples are hashable in Python, which makes them valid keys for dictionaries. This is because tuples are immutable, meaning their contents cannot be changed after they are created. Hashable objects, like tuples, have a __hash__()
method that allows Python to generate a unique hash value, making them suitable for use as dictionary keys.
# Using a tuple as a key in a dictionary
coordinates = {}
# Adding a tuple key
coordinates[(10, 20)] = "Point A"
coordinates[(30, 40)] = "Point B"
# Accessing values using tuple keys
print(coordinates[(10, 20)]) # Output: Point A
print(coordinates[(30, 40)]) # Output: Point B
PythonIn this example, (10, 20)
and (30, 40)
are tuples used as keys in the coordinates
dictionary. You can use these keys to retrieve the associated values.
However, it’s important to remember that only immutable tuples (i.e., those containing immutable elements like strings, numbers, or other tuples) can be used as dictionary keys. If a tuple contains mutable elements like lists, it won’t be hashable and cannot be used as a key.
Functions that return multiple values can use tuples
In Python, a function can return multiple values using tuples. When you return multiple values separated by commas, Python automatically packs them into a tuple. The caller can then unpack these values into separate variables.
def get_coordinates():
x = 10
y = 20
return x, y
coordinates = get_coordinates()
print(coordinates) # Output: (10, 20)
print(type(coordinates)) # <class 'tuple'>
# Unpacking the tuple into separate variables
x_coord, y_coord = get_coordinates()
print(x_coord) # Output: 10
print(y_coord) # Output: 20
PythonUnderlying data structures
Data Structure | Underlying Structure |
---|---|
List | Dynamic Array |
Tuple | Static Array |
Set | Hash Table |
Dictionary | Hash Table |
Use case
Sets
- Removing duplicates from a list.
- Checking membership and performing set operations like union, intersection, and difference.
Dictionaries
- Storing and retrieving data by keys.
- Implementing lookups, caching, and associative arrays.
- Representing JSON-like data structures.
Tuples
- Storing fixed collections of items where immutability is required.
- Using tuples as keys in dictionaries (since they are washable).
- Returning multiple values from a function.
List
- Storing and manipulating collections of items.
- Iterating over elements to perform operations.
- Implementing stacks and queues.
Example
Healthcare System
List:
Patient Appointments: A list to manage daily appointments at a clinic. Each patient’s appointment is an item on the list.
appointments = ['John Doe - 10:00 AM', 'Jane Smith - 10:30 AM', 'Alice Johnson - 11:00 AM']
PythonDictionaries:
Patient Records: A dictionary where each patient’s ID is the key, and their medical history, contact details, and prescriptions are the values.
patient_records = {
'P001': {'name': 'John Doe', 'age': 45, 'conditions': ['Diabetes'], 'medications': ['Metformin']},
'P002': {'name': 'Jane Smith', 'age': 30, 'conditions': ['Hypertension'], 'medications': ['Lisinopril']}
}
PythonTuples
Lab Test Results: A tuple to store immutable data such as the results of a specific lab test (e.g., cholesterol levels).
cholesterol_test = ('Cholesterol', 180, 'mg/dL')
PythonSet
Unique Medications: A set to manage a collection of unique medications prescribed at the clinic to avoid duplicates.
prescribed_medications = {'Metformin', 'Lisinopril', 'Atorvastatin'}
PythonE-commerce Platform
Lists:
Shopping Cart: A list to store items a user intends to purchase. Each item is an entry in the list.
shopping_cart = ['Laptop', 'Wireless Mouse', 'Keyboard']
PythonDictionaries:
Product Catalog: A dictionary where product IDs are keys and the corresponding product details (name, price, stock) are values.
product_catalog = {
'P001': {'name': 'Laptop', 'price': 999.99, 'stock': 20},
'P002': {'name': 'Wireless Mouse', 'price': 19.99, 'stock': 150}
}
PythonTuples:
Customer Order: A tuple to store order details that should not change, like the order number and date.
order_details = ('Order123', '2024-08-29')
PythonSets:
Unique Customers: A set to track unique customers who have purchased, ensuring no duplicate entries.
unique_customers = {'Customer001', 'Customer002', 'Customer003'}
PythonEducational System
Lists
Classroom Attendance: A list to keep track of students present in a classroom.
attendance_list = ['Alice', 'Bob', 'Charlie']
PythonDictionaries
Grades Book: A dictionary where each student’s name is the key and their grades are the values.
grades = {
'Alice': {'Math': 95, 'Science': 88},
'Bob': {'Math': 78, 'Science': 82},
'Charlie': {'Math': 85, 'Science': 91}
}
PythonTuples:
Course Details: A tuple to store unchangeable course information like course ID and title.
course_details = ('CS101', 'Introduction to Computer Science')
PythonSets:
Extracurricular Participants: A set to manage students participating in extracurricular activities, ensuring each student is listed only once.
extracurricular_participants = {'Alice', 'David', 'Eva'}
PythonSocial Media Platform
Lists:
User’s Friends: A list to maintain the order of friends in a user’s friend list.
friends_list = ['Alice', 'Bob', 'Charlie']
PythonDictionaries:
User Profiles: A dictionary where each user’s username is the key and their profile information (name, age, bio) is the value.
user_profiles = {
'user1': {'name': 'Alice', 'age': 28, 'bio': 'Love traveling and photography'},
'user2': {'name': 'Bob', 'age': 34, 'bio': 'Avid reader and gamer'}
}
PythonTuples:
Post Metadata: A tuple to store immutable metadata about a post, such as post ID and timestamp.
post_metadata = ('Post123', '2024-08-29 10:15:00')
PythonSets:
Liked Posts: A set to manage posts that a user has liked, ensuring no duplicate likes.
liked_posts = {'Post123', 'Post456', 'Post789'}
PythonMembership check
A membership check refers to the process of verifying whether a particular element (or value) exists within a given collection or data structure.
Code
# Data structures
my_list = [1, 2, 3, 4, 5]
my_set = {1, 2, 3, 4, 5}
my_dict = {'a': 1, 'b': 2, 'c': 3}
my_tuple = (1, 2, 3, 4, 5)
# Membership checks
value_to_check = 3
key_to_check = 'b'
# Check in list
if value_to_check in my_list:
print(f"{value_to_check} is in the list!")
else:
print(f"{value_to_check} is not in the list!")
# Check in set
if value_to_check in my_set:
print(f"{value_to_check} is in the set!")
else:
print(f"{value_to_check} is not in the set!")
# Check in dictionary (for key)
if key_to_check in my_dict:
print(f"'{key_to_check}' is a key in the dictionary!")
else:
print(f"'{key_to_check}' is not a key in the dictionary!")
# Check in tuple
if value_to_check in my_tuple:
print(f"{value_to_check} is in the tuple!")
else:
print(f"{value_to_check} is not in the tuple!")
PythonOutput
3 is in the list!
3 is in the set!
'b' is a key in the dictionary!
3 is in the tuple!
PythonPoints to be noted
- Set: Fastest for membership checks (average O(1)).
- Dictionary: Also fast for key membership checks (O(1) on average).
- List: Slower for membership checks (O(n)).
- Tuple: Same as lists (O(n)).
Set is preferred for a membership check
Summary:
- Set: Best for unique items, and fast membership checking (e.g., removing duplicate emails).
- Dictionary: Best for key-value pairs, and fast lookups (e.g., storing student grades, storing survey responses).
- Tuple: Best for immutable data, fixed values (e.g., storing event timestamps, tracking user activity).
- List: Best for ordered, modifiable data (e.g., managing playlists, logging events, sorting products).
Conclusion
Python’s data structures—lists, dictionaries, sets, and tuples—each have unique characteristics and use cases. Lists and dictionaries are mutable, making them ideal for dynamic data. Sets provide unique, unordered collections optimized for mathematical operations. Tuples, with their immutability, are perfect for storing related data that should not change. Understanding these data structures will greatly enhance your ability to write efficient and effective Python code.
2 thoughts on “Mastering Python: Lists, Dictionaries, Tuples, and Sets”