Git Rebase and git merge are two common approaches for integrating feature branches back into the main codebase or consolidating branches. When multiple people work on separate branches, differences can arise between them. Rebasing or merging helps bring these changes together into a single branch.
Table of Contents
What is Branching?
Branching means splitting from the master branch to work separately without affecting the main code and other developers.

Managing branches and combining branches is a central part of any collaborative project.
Two common approaches for merging two branches or integrating feature branches back into the main codebase are git merge and git rebase.
- git merge
- git rebase
Each method has distinct advantages and disadvantages, and understanding when to use each can make a big difference in the clarity and maintainability of your project’s history.
What is Git Rebase?
Git rebase provides a way to incorporate changes from one branch into another by moving or “replaying” the commits. With rebasing, Git changes the base of your branch so it appears as though your work started on the latest commit of the mainline branch. Git rebasing looks as follows:
feature(branch)> git rebase Main
Python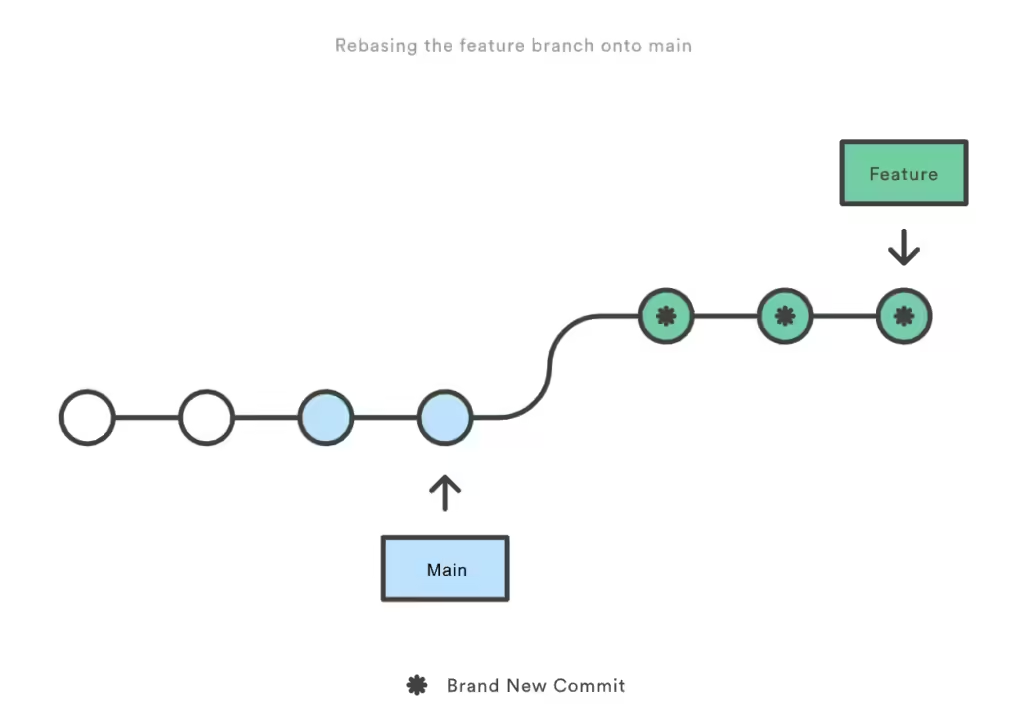
Advantages of Git Rebase
- Cleaner History: Rebase creates a linear, more readable commit history. This makes it easier to see the progression of changes.
- Ideal for Local Work: Rebasing is often used in local branches before pushing changes to a shared repository. It helps keep your work isolated and clean.
- Avoids Merge Commits: Rebase eliminates unnecessary merge commits, especially helpful for long-lived feature branches.
Disadvantages of Git Rebase
- Risk of Losing Changes: If you’re not careful, rebasing can inadvertently drop or modify commits, especially when working on shared branches.
- Conflicts Can Be Tricky: Since rebase replays every commit, conflicts need to be resolved one commit at a time. This can be tedious if you’re working with a large number of commits.
- Modifies History: Because rebasing rewrites history, it’s best used only for local branches. Rebasing a shared branch can cause confusion for other team members.
What has changed?
When you run git rebase Main on your feature branch, the commit history of the feature branch (feature(branch)) is modified.
Here’s a breakdown of what changes:
- Feature Branch Commits: The commits on the feature(branch) are replayed on top of the latest commit in Main, essentially rewriting their history to appear as if they were created after the latest Main branch commits.
- Commit IDs: Since each commit in Git is identified by a unique hash, replaying the commits on top of Main changes their hash IDs, making these commits appear “new” even though their content is the same.
- Main Branch Commits: The commits on Main remain completely unchanged. Only the feature branch’s history is rewritten to incorporate the latest state of Main.
In summary:
Changed history: feature(branch) (rebased onto Main)
Unchanged history: Main
Is one of the branches deleted?
After performing a rebase, you still have both branches (Main and feature(branch)) in your repository. The rebase operation does not delete any branches; it only changes the commit history of the branch you’re rebasing (in this case, feature(branch)) to appear as if it was developed on top of the Main branch.
Here’s what each branch represents post-rebase:
- Main Branch: Remains unchanged and still contains the original commits up to its latest commit.
- Feature branch: Now includes all commits from Main, followed by any additional commits you made on the feature(branch).
The rebase process just makes your feature branch up to date with Main, but both branches still exist independently.
Effect of rebase on different branches
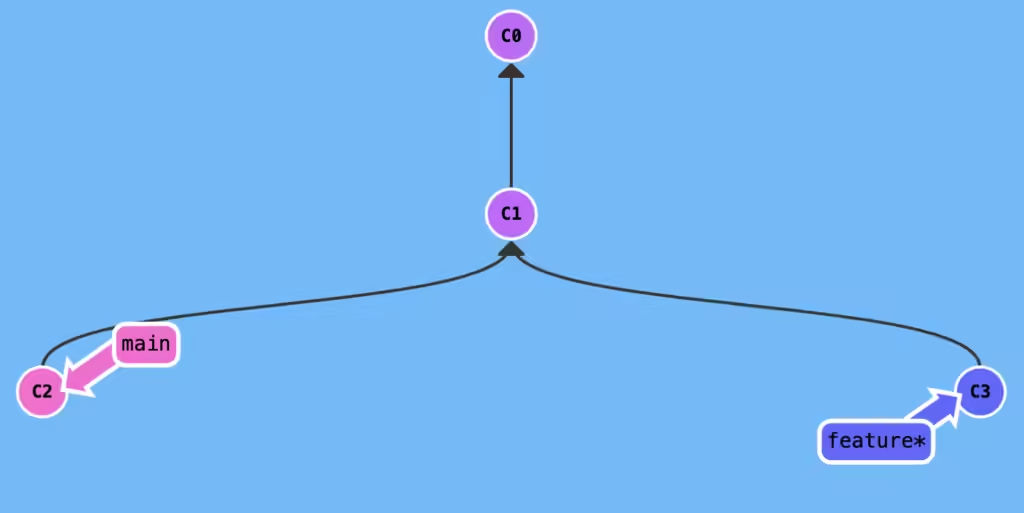
Case 1: Inside the feature branch, we rebase the Main
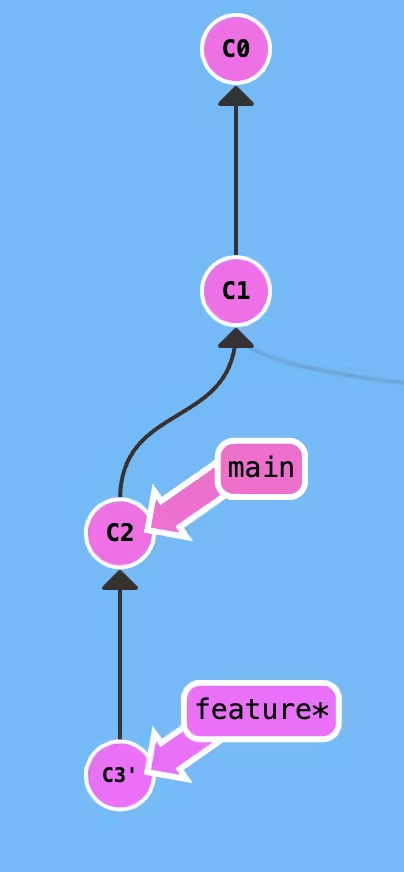
feature(branch)> git rebase Main
Python- Commits from the feature branch replay over the Master branch
- Both branch reference is pointing at a different position
Case 2: Inside the Main branch, we rebase the feature

Main(branch)> git rebase feature
Python- Commits from the Main branch replay over the feature branch
- Both branch reference is pointing at a different position
Git Merge
Git merge is a straightforward approach to combining the work done in two branches. When you merge a branch into another, Git creates a new “merge commit” in the destination branch. This merge commit brings together the history from both branches without altering the history of either branch.
feature(branch)> git merge Main
Python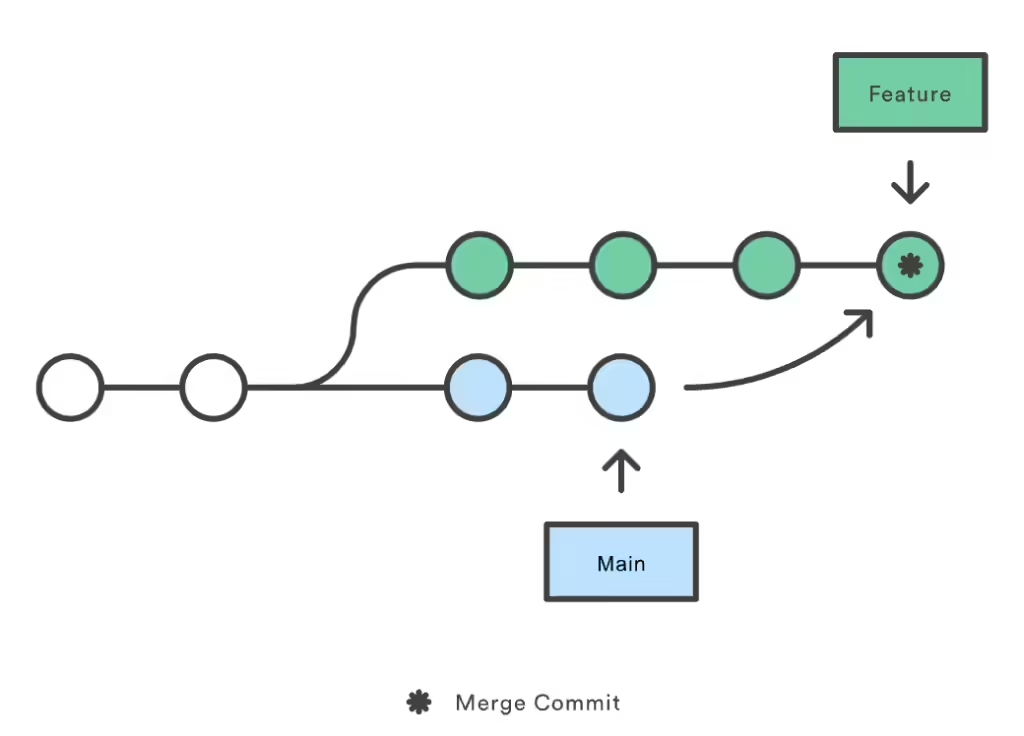
Where is the new commit?
When you run the command git merge Main from the feature branch, Git will attempt to merge the Main branch into your current branch (which is a feature). If there are new commits in the Main branch that are not yet in the feature, Git will include those commits in the merge.
After the merge, you will have:
- Commits from Main: Any new commits in the Main branch that were not in the feature will be merged into the feature branch.
- New commit on the feature: Git will create a new “merge commit” on the feature branch that combines the histories of both branches.
- Changes from the Main branch are merged with the feature branch
- Changes from the feature branch are not there in the main branch
Once the merge is complete, a new commit will appear on the feature branch (assuming no fast-forward merge).
Advantages of Git Merge
- Preserves History: Git merge maintains a clear record of all the commits in both branches. You can see exactly where and when changes occurred.
- Useful for Collaboration: The linear history with merge commits shows when two branches were integrated. This is especially useful in team projects.
- Easier Conflict Resolution: With each branch’s changes clearly visible, resolving conflicts in a merge is generally straightforward.
Disadvantages of Git Merge
- Cluttered History: Merges can make your commit history more complex, with multiple merge commits that might make it harder to follow a linear progression.
- Harder to Read History in Long Projects: In a long-running project with frequent merges, you might end up with a complex web of branches and merges, which can be difficult to understand at a glance.
When to Use Git Merge vs. Git Rebase
Both merge and rebase have appropriate use cases. Here are some guidelines to help you decide:
Use Git Merge:
- When working in a team environment with multiple collaborators.
- For merging long-lived branches where preserving history is important.
- To avoid history rewriting, especially on branches that others are working on.
Use Git Rebase:
- For local branches, where you’re the only one working on a feature.
- To create a clean, linear history before integrating your feature into the main branch.
- When you need to keep your branch up-to-date with the mainline without introducing merge commits.
The Golden Rule of Git Rebase
One key rule for rebasing: never rebase commits that have been pushed to a shared or public repository. Since rebasing changes commit history, it can create issues for collaborators who are working with the same branch.
Why?
- Breaks Others’ Work
Git relies on commit hashes to track changes. When you rebase, the commits are rewritten, meaning their hashes change. If other team members have already pulled or based work on the original commits, rebasing will cause conflicts in their histories. They will end up with broken references and need to perform complex recovery steps to synchronize their work with the rewritten history. - Loss of Shared Context
Rebase changes the commit history by flattening or reordering commits. This can lead to losing the shared context of when and why certain decisions were made. When working collaboratively, it’s important to preserve the exact sequence of commits so that everyone involved can track the full history of the project. - Conflicts and Confusion
After rebasing, other collaborators will have to deal with a situation where their local histories don’t align with the rewritten remote history. This can cause confusion and errors, especially when resolving merge conflicts. - Potential for Data Loss
If someone hasn’t yet pulled or saved the old commits, there’s a risk of losing their work after you rebase and push the changes, as the old commit history is no longer valid.
Best Practices:
- Avoid rebasing shared commits: As a general rule, only rebase local changes that haven’t been shared yet. Once commits are pushed to the shared repository, use merge instead to avoid disrupting the work of others.
- Use rebasing for cleanup: You can use rebase to clean up your own feature branches before pushing to the shared repository, but only after making sure others aren’t depending on those commits.
Never rebase commits that have been pushed to a shared repository because it alters history in a way that can confuse collaborators and cause conflicts in the codebase. Instead, use merge to combine changes without rewriting history.
Conclusion
Understanding the differences between git merge and git rebase is crucial for effective version control. While merge is ideal for preserving history and collaboration, rebase provides a way to streamline and simplify your commit history. By mastering both, you can create a workflow that suits your project’s needs and collaborate more effectively.
1 thought on “Choosing Between Git Rebase and Merge”